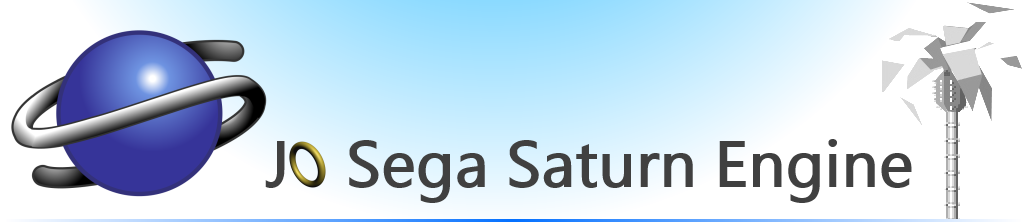 |
Jo Engine
2024.04.28
Jo Sega Saturn Engine
|
Go to the documentation of this file.
39 #ifdef JO_COMPILE_WITH_3D_SUPPORT
42 # define JO_3D_PLANE_VERTICES(SIZE) \
44 { -SIZE, -SIZE, 0 }, \
51 # define JO_3D_TRIANGLE_VERTICES(SIZE) \
53 { -SIZE, -SIZE, 0 }, \
59 # define JO_3D_CUBE_VERTICES(SIZE) \
61 { -SIZE, -SIZE, SIZE }, \
62 { SIZE, -SIZE, SIZE }, \
63 { SIZE, SIZE, SIZE }, \
64 { -SIZE, SIZE, SIZE }, \
65 { -SIZE, -SIZE, -SIZE }, \
66 { -SIZE, -SIZE, SIZE }, \
67 { -SIZE, SIZE, SIZE }, \
68 { -SIZE, SIZE, -SIZE }, \
69 { SIZE, -SIZE, -SIZE }, \
70 { -SIZE, -SIZE, -SIZE }, \
71 { -SIZE, SIZE, -SIZE }, \
72 { SIZE, SIZE, -SIZE }, \
73 { SIZE, -SIZE, SIZE }, \
74 { SIZE, -SIZE, -SIZE }, \
75 { SIZE, SIZE, -SIZE }, \
76 { SIZE, SIZE, SIZE }, \
77 { -SIZE, -SIZE, -SIZE }, \
78 { SIZE, -SIZE, -SIZE }, \
79 { SIZE, -SIZE, SIZE }, \
80 { -SIZE, -SIZE, SIZE }, \
81 { -SIZE, SIZE, SIZE }, \
82 { SIZE, SIZE, SIZE }, \
83 { SIZE, SIZE, -SIZE }, \
84 { -SIZE, SIZE, -SIZE } \
125 #if JO_COMPILE_USING_SGL
187 slLookAt(cam->viewpoint, cam->target, cam->
z_angle);
206 extern Uint16 TotalPolygons;
207 return TotalPolygons;
216 extern Uint16 TotalVertices;
217 return TotalVertices;
226 extern Uint16 DispPolygons;
373 for (
unsigned int i = 0; i < mesh->
data.nbPolygon; ++i)
416 mesh->
data.pntbl[index][0] = x;
417 mesh->
data.pntbl[index][1] = y;
418 mesh->
data.pntbl[index][2] = z;
430 mesh->
data.pltbl[index].norm[0] = x;
431 mesh->
data.pltbl[index].norm[1] = y;
432 mesh->
data.pltbl[index].norm[2] = z;
704 slTranslate(toFIXED(x), toFIXED(y), toFIXED(z));
714 slTranslate(x, y, z);
766 slPutPolygon(&quad->
data);
774 slPutPolygon(&mesh->
data);
783 return mesh->
data.nbPolygon;
808 while (--array_size >= 0)
jo_3d_draw(array + array_size);
891 slScale(toFIXED(x), toFIXED(y), toFIXED(z));
941 static __jo_force_inline bool jo_3d_window(
const int left,
const int top,
const int right,
const int bottom,
const int z_limit,
const int center_x,
const int center_y)
943 return slWindow(left, top, right, bottom, z_limit, center_x, center_y) != 0;
952 if (angle < 10 || angle > 160)
static __jo_force_inline void jo_3d_display_level(const unsigned short level)
Set the display level of the viewing volume.
Definition: 3d.h:961
Vector for 3D computation using integer.
Definition: types.h:142
static __jo_force_inline void jo_3d_set_palette(jo_3d_quad *const quad, const int palette_id)
Set a palette on the quadrilateral.
Definition: 3d.h:381
static __jo_force_inline void jo_3d_rotate_matrix(short x, short y, short z)
Rotate 3D matrix using degree.
Definition: 3d.h:620
static __jo_force_inline void jo_3d_set_transparency(jo_3d_quad *const quad, const bool enabled)
Enable/Disable transparency on the quadrilateral.
Definition: 3d.h:508
static __jo_force_inline unsigned int jo_3d_get_polygon_count(void)
Get polygon count (visible and not visible)
Definition: 3d.h:204
static __jo_force_inline void jo_3d_rotate_matrix_rad_x(float x)
Rotate 3D matrix using radian (X axis)
Definition: 3d.h:666
static __jo_force_inline unsigned int jo_3d_get_displayed_polygon_count(void)
Get displayed polygon count.
Definition: 3d.h:224
static __jo_force_inline void jo_3d_light(const int x, const int y, const int z)
Use a light source.
Definition: 3d.h:450
static __jo_force_inline void jo_3d_camera_look_at(jo_camera *const cam)
look at the camera
Definition: 3d.h:185
#define jo_core_error(...)
Definition: core.h:45
static __jo_force_inline void jo_3d_translate_matrix_y(int y)
Translate 3D matrix (Y axis)
Definition: 3d.h:738
static __jo_force_inline void jo_3d_translate_matrix_fixed(jo_fixed x, jo_fixed y, jo_fixed z)
Translate 3D matrix.
Definition: 3d.h:712
static __jo_force_inline void jo_3d_translate_matrixf(float x, float y, float z)
Translate 3D matrix (using floating numbers)
Definition: 3d.h:702
void jo_3d_set_mesh_screen_doors(jo_3d_mesh *const mesh, const bool enabled)
Enable/Disable screen doors transparency on the mesh.
static __jo_force_inline void jo_3d_translate_matrix(int x, int y, int z)
Translate 3D matrix.
Definition: 3d.h:722
static __jo_force_inline void jo_3d_rotate_matrix_x(short x)
Rotate 3D matrix using degree (X axis)
Definition: 3d.h:630
void jo_3d_set_mesh_texture(jo_3d_mesh *const mesh, const int sprite_id)
Set a texture on the mesh.
static __jo_force_inline void jo_3d_set_mesh_normal(jo_3d_mesh *const mesh, const jo_fixed x, const jo_fixed y, const jo_fixed z, const unsigned int index)
Set quad normal in mesh.
Definition: 3d.h:428
jo_sprite_attributes __jo_sprite_attributes
Current displayed sprite attribute (internal engine usage)
static __jo_force_inline void jo_3d_set_mesh_vertice(jo_3d_mesh *const mesh, const jo_fixed x, const jo_fixed y, const jo_fixed z, const unsigned int index)
Set vertice position in mesh.
Definition: 3d.h:414
static __jo_force_inline bool jo_3d_set_sprite_palette(const int sprite_id, const int palette_id)
Set a palette on a sprite (3D)
Definition: 3d.h:391
void jo_3d_set_mesh_polygon_light(jo_3d_mesh *const mesh, const bool enabled, const unsigned int index)
Enable/Disable light on one polygon on the mesh.
static __jo_force_inline void jo_3d_camera_set_viewpoint(jo_camera *const cam, const int x, const int y, const int z)
Set the viewpoint of the camera (where the cameraman is)
Definition: 3d.h:143
static __jo_force_inline void jo_3d_translate_matrix_z(int z)
Translate 3D matrix (Z axis)
Definition: 3d.h:746
static __jo_force_inline void jo_3d_camera_init(jo_camera *const cam)
Initialize the camera with default values.
Definition: 3d.h:175
static __jo_force_inline ANGLE jo_DEGtoANG_int(const int deg)
Replacement for DEGtoANG using interger.
Definition: math.h:650
jo_pos3D jo_vertice
Vertice structure.
Definition: 3d.h:88
#define JO_MULT_BY_65536(X)
Multiply a variable by 65536.
Definition: math.h:138
void jo_3d_set_mesh_light(jo_3d_mesh *const mesh, const bool enabled)
Enable/Disable light on the mesh.
static __jo_force_inline void jo_3d_draw_list(jo_list *const list)
Draw a list of quadrilateral.
Definition: 3d.h:797
static __jo_force_inline jo_3d_mesh * jo_3d_create_mesh(const unsigned int quad_count)
Create a mesh programmatically from vertices.
Definition: 3d.h:272
static __jo_force_inline void jo_3d_camera_set_target(jo_camera *const cam, const int x, const int y, const int z)
Set the target of the camera (where the scene is located)
Definition: 3d.h:156
static __jo_force_inline void jo_3d_rotate_matrix_rad_z(float z)
Rotate 3D matrix using radian (Z axis)
Definition: 3d.h:682
static __jo_force_inline jo_3d_mesh * jo_3d_create_mesh_from_vertices(const unsigned int quad_count, jo_vertice *const vertices)
Create a mesh programmatically from vertices.
Definition: 3d.h:262
void jo_sprite_draw(const int sprite_id, const jo_pos3D *const pos, const bool centered_style_coordinates, const bool billboard)
(internal engine usage)
static __jo_force_inline void jo_3d_set_light(jo_3d_quad *const quad, const bool enabled)
Enable/Disable light on the quad.
Definition: 3d.h:476
#define JO_MULT_BY_256(X)
Multiply a variable by 256.
Definition: math.h:108
jo_fixed fixed_scale_y
Definition: types.h:272
jo_list_data data
Definition: list.h:67
unsigned short jo_color
15 bits color type
Definition: types.h:42
ANGLE z_angle
Definition: 3d.h:103
static __jo_force_inline void jo_3d_rotate_matrix_rad_y(float y)
Rotate 3D matrix using radian (Y axis)
Definition: 3d.h:674
static __jo_force_inline void jo_3d_rotate_matrix_y(short y)
Rotate 3D matrix using degree (Y axis)
Definition: 3d.h:638
static __jo_force_inline void jo_3d_mesh_draw(jo_3d_mesh *const mesh)
Draw a mesh.
Definition: 3d.h:772
#define __jo_force_inline
force inline attribute (and prevent Doxygen prototype parsing bug)
Definition: types.h:39
int jo_fixed
Fixed point Q16.16 number.
Definition: types.h:49
static __jo_force_inline unsigned int jo_3d_get_vertices_count(void)
Get vertice count.
Definition: 3d.h:214
static __jo_force_inline void jo_3d_translate_matrix_x(int x)
Translate 3D matrix (X axis)
Definition: 3d.h:730
static __jo_force_inline void jo_3d_draw_sprite_at(const int sprite_id, const int x, const int y, const int z)
Draw a sprite.
Definition: 3d.h:828
static __jo_force_inline bool jo_3d_window(const int left, const int top, const int right, const int bottom, const int z_limit, const int center_x, const int center_y)
Sets up a window region on the projection surface.
Definition: 3d.h:941
static __jo_force_inline jo_fixed jo_int2fixed(const int x)
Convert int to jo engine fixed.
Definition: math.h:415
void jo_3d_create_plane(jo_3d_quad *const quad, jo_vertice *const vertices)
Create a four vertices polygon (Quadrilateral shape)
static __jo_force_inline void jo_list_foreach(jo_list *const list, jo_node_callback callback)
Iterate on the list.
Definition: list.h:221
ATTR attribute
Definition: 3d.h:110
static __jo_force_inline bool jo_3d_draw_sprite(const int sprite_id)
Draw a sprite.
Definition: 3d.h:815
bool jo_3d_create_sprite_quad(const int sprite_id)
void jo_3d_create_cube(jo_3d_quad *array, jo_vertice *const vertices)
Create a cube.
static __jo_force_inline void jo_3d_set_scale(const int x, const int y, const int z)
Change scale.
Definition: 3d.h:899
List struct.
Definition: list.h:74
Camera structure.
Definition: 3d.h:92
void jo_3d_set_mesh_polygon_transparency(jo_3d_mesh *const mesh, const bool enabled, const unsigned int index)
Enable/Disable transparency on one polygon on the mesh.
Quadrilateral shape structure.
Definition: 3d.h:108
PDATA data
Definition: 3d.h:116
Mesh structure.
Definition: 3d.h:115
static __jo_force_inline void jo_3d_set_screen_doors(jo_3d_quad *const quad, const bool enabled)
Enable/Disable screen doors transparency on the quad.
Definition: 3d.h:534
void jo_3d_set_mesh_polygon_color_ex(jo_3d_mesh *const mesh, const jo_color color, const unsigned int index, bool wireframe)
Set the color on one polygon on the mesh with wireframe option.
3D position
Definition: types.h:69
void jo_3d_free_sprite_quad(const int sprite_id)
Delete sprite quad.
void jo_3d_free_mesh(const jo_3d_mesh *const mesh)
Free a mesh created with jo_3d_create_mesh()
Node struct.
Definition: list.h:66
PDATA data
Definition: 3d.h:109
static __jo_force_inline unsigned int jo_3d_get_mesh_polygon_count(jo_3d_mesh *const mesh)
Get polygon count on the mesh.
Definition: 3d.h:781
static __jo_force_inline void jo_3d_set_mesh_palette(jo_3d_mesh *const mesh, const int palette_id)
Set a palette on one polygon on the mesh.
Definition: 3d.h:371
static __jo_force_inline void jo_3d_push_matrix(void)
Push 3D matrix.
Definition: 3d.h:593
static __jo_force_inline void jo_3d_restore_scale(void)
Restore default scale for every 3d model displayed after this call.
Definition: 3d.h:916
static __jo_force_inline void jo_3d_pop_matrix(void)
Pop 3D matrix.
Definition: 3d.h:600
static __jo_force_inline void jo_3d_set_texture(jo_3d_quad *const quad, const int sprite_id)
Set a texture on the quadrilateral.
Definition: 3d.h:341
jo_fixed fixed_scale_x
Definition: types.h:271
void jo_3d_create_triangle(jo_3d_quad *const quad, jo_vertice *const vertices)
Create a triangle.
static __jo_force_inline void jo_3d_draw_billboard(const int sprite_id, const int x, const int y, const int z)
Draw billboard.
Definition: 3d.h:842
jo_3d_quad * __jo_sprite_quad[JO_MAX_SPRITE]
Sprite quads (internal engine usage)
void jo_3d_set_mesh_transparency(jo_3d_mesh *const mesh, const bool enabled)
Enable/Disable transparency on the mesh.
static __jo_force_inline void __internal_jo_sprite_set_position3D(const int x, const int y, const int z)
Internal usage don't use it.
Definition: sprites.h:69
static __jo_force_inline jo_3d_quad * jo_3d_get_sprite_quad(const int sprite_id)
Get sprite quad.
Definition: 3d.h:304
void jo_3d_set_mesh_color(jo_3d_mesh *const mesh, const jo_color color)
Set the color on the mesh.
void jo_3d_set_mesh_polygon_texture(jo_3d_mesh *const mesh, const int sprite_id, const unsigned int index)
Set a texture on one polygon on the mesh.
static __jo_force_inline void jo_3d_set_mesh_polygon_palette(jo_3d_mesh *const mesh, const int palette_id, const unsigned int index)
Set a palette on the mesh.
Definition: 3d.h:362
static __jo_force_inline void jo_3d_set_scale_fixed(const jo_fixed x, const jo_fixed y, const jo_fixed z)
Change scale using fixed number.
Definition: 3d.h:909
static __jo_force_inline void jo_3d_draw_array(jo_3d_quad *const array, int array_size)
Draw an array of quadrilateral.
Definition: 3d.h:806
jo_3d_mesh * jo_3d_create_mesh_from_vertices_and_normals(const unsigned int quad_count, jo_vertice *const vertices, jo_vector *const normals)
Create a mesh programmatically from vertices.
static __jo_force_inline void jo_3d_set_color(jo_3d_quad *const quad, const jo_color color)
Set the color on the quadrilateral.
Definition: 3d.h:577
static __jo_force_inline void jo_3d_set_mesh_polygon_color(jo_3d_mesh *const mesh, const jo_color color, const unsigned int index)
Set the color on one polygon on the mesh.
Definition: 3d.h:562
static __jo_force_inline void jo_3d_draw_scaled_billboard(const int sprite_id, const int x, const int y, const int z, const int scale)
Draw billboard with a specific scale.
Definition: 3d.h:856
static __jo_force_inline void jo_3d_rotate_matrix_rad(float x, float y, float z)
Rotate 3D matrix using radian.
Definition: 3d.h:656
static __jo_force_inline void jo_3d_rotate_matrix_z(short z)
Rotate 3D matrix using degree (Z axis)
Definition: 3d.h:646
void * ptr
Definition: list.h:59
static __jo_force_inline void jo_3d_camera_set_z_angle(jo_camera *const cam, const int angle)
Set the angle of the camera.
Definition: 3d.h:167
static __jo_force_inline void jo_3d_set_scalef(const float x, const float y, const float z)
Change scale using floating number.
Definition: 3d.h:889
static __jo_force_inline void jo_3d_draw(jo_3d_quad *const quad)
Draw a quadrilateral.
Definition: 3d.h:764
void jo_3d_set_mesh_polygon_wireframe(jo_3d_mesh *const mesh, const unsigned int index, bool wireframe)
Set wireframe mode on the mesh.
void jo_3d_set_mesh_polygon_screen_doors(jo_3d_mesh *const mesh, const bool enabled, const unsigned int index)
Enable/Disable screen doors transparency on one polygon on the mesh.
#define JO_NO_ZOOM
Value used to keep the original scale.
Definition: sprites.h:314
static __jo_force_inline void jo_3d_perspective_angle(const int angle)
Set the perspective angle.
Definition: 3d.h:949
static __jo_force_inline void __jo_poylgon_draw_iterator(jo_node *node)
Internal usage for polygon display.
Definition: 3d.h:789