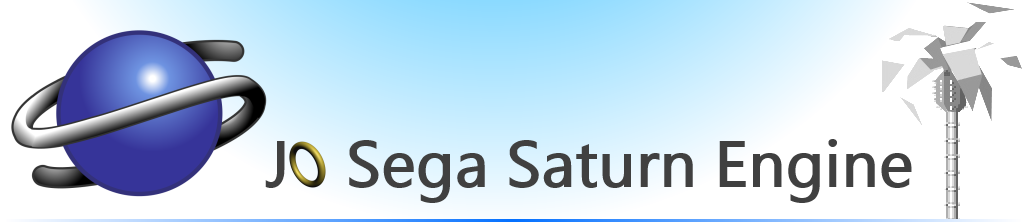 |
Jo Engine
2024.04.28
Jo Sega Saturn Engine
|
Go to the documentation of this file.
38 #ifdef JO_COMPILE_WITH_FS_SUPPORT
44 # define JO_ROOT_DIR ((const char *)0)
46 # define JO_CURRENT_DIR ((const char *)0)
48 # define JO_PARENT_DIR ("..")
void jo_fs_close(jo_file *const file)
Close a file.
bool jo_fs_open(jo_file *const file, const char *const filename)
Open a file.
char * jo_fs_read_file_in_dir(const char *const filename, const char *const sub_dir, int *len)
Read a file on the CD.
bool jo_fs_seek_forward(jo_file *const file, unsigned int nbytes)
Seek forward from current position of a file.
static __jo_force_inline bool jo_fs_read_file_async(const char *const filename, jo_fs_async_read_callback callback, int optional_token)
Read a file on the CD asynchronously.
Definition: fs.h:102
#define __jo_force_inline
force inline attribute (and prevent Doxygen prototype parsing bug)
Definition: types.h:39
int jo_fs_read_next_bytes(jo_file *const file, char *buffer, unsigned int nbytes)
Read bytes from a file.
bool jo_fs_read_file_async_ptr(const char *const filename, jo_fs_async_read_callback callback, int optional_token, void *buf)
Read a file on the CD asynchronously and put the contents to "buf".
void jo_fs_cd(const char *const sub_dir)
Change the current directory (equivalent of Unix cd command)
static __jo_force_inline char * jo_fs_read_file(const char *const filename, int *len)
Read a file on the CD.
Definition: fs.h:82
void(* jo_fs_async_read_callback)(char *contents, int length, int optional_token)
Function prototype for ()
Definition: fs.h:54
File definition.
Definition: types.h:291
char * jo_fs_read_file_ptr(const char *const filename, void *buf, int *len)
Read a file on the CD and put the contents to "buf".