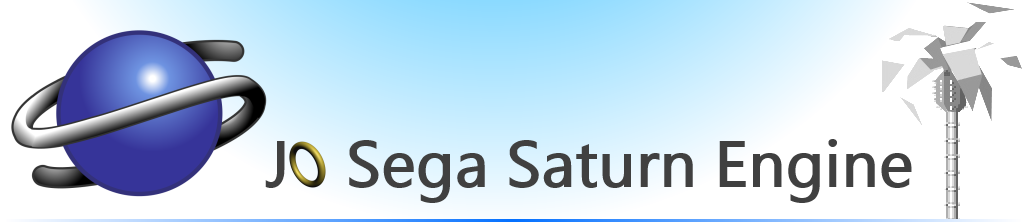 |
Jo Engine
2024.04.28
Jo Sega Saturn Engine
|
Go to the documentation of this file.
34 #ifndef __JO_HITBOX_H__
35 # define __JO_HITBOX_H__
static __jo_force_inline bool jo_hitbox_detection_custom_boundaries(const int sprite_id1, const int x1, const int y1, const int x2, const int y2, const int w2, const int h2)
Fast method to get if the sprite intersects with the box (HitBox processing)
Definition: hitbox.h:69
#define JO_DIV_BY_2(X)
Devide a variable by 2.
Definition: math.h:144
static __jo_force_inline bool jo_hitbox_detection(const int sprite_id1, const int x1, const int y1, const int sprite_id2, const int x2, const int y2)
Fast method to get if two sprites intersects (HitBox processing)
Definition: hitbox.h:46
static __jo_force_inline bool jo_square_intersect(const int x1, const int y1, const int w1, const int h1, const int x2, const int y2, const int w2, const int h2)
Fast method to get if two square intersects (HitBox processing)
Definition: math.h:1747
#define __jo_force_inline
force inline attribute (and prevent Doxygen prototype parsing bug)
Definition: types.h:39
jo_texture_definition __jo_sprite_def[JO_MAX_SPRITE]
(internal engine usage)