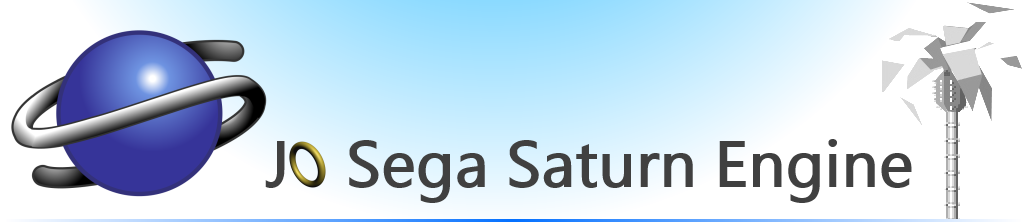 |
Jo Engine
2024.04.28
Jo Sega Saturn Engine
|
Go to the documentation of this file.
35 #ifndef __JO_KEYBOARD_H__
36 # define __JO_KEYBOARD_H__
38 #ifdef JO_COMPILE_WITH_KEYBOARD_SUPPORT
67 # define __JO_KEYBOARD_MAPPING_SIZE (128)
106 PerKeyBoard *keyboard;
108 keyboard = (PerKeyBoard *)&Smpc_Peripheral[0];
111 if (keyboard->cond & PER_KBD_CL)
char __internal_keyboard_caps_lock_mapping[]
Keyboard mapping (internal engine usage)
#define __JO_KEYBOARD_MAPPING_SIZE
Keyboard mapping size (internal engine usage)
Definition: keyboard.h:67
@ JO_KEYBOARD_LEFT
Definition: keyboard.h:78
@ JO_KEYBOARD_F9
Definition: keyboard.h:96
@ JO_KEYBOARD_HOME
Definition: keyboard.h:86
@ JO_KEYBOARD_DELETE
Definition: keyboard.h:85
@ JO_KEYBOARD_F6
Definition: keyboard.h:93
@ JO_KEYBOARD_F11
Definition: keyboard.h:98
@ JO_KEYBOARD_RIGHT
Definition: keyboard.h:77
@ JO_KEYBOARD_TAB
Definition: keyboard.h:84
@ JO_KEYBOARD_F5
Definition: keyboard.h:92
#define __jo_force_inline
force inline attribute (and prevent Doxygen prototype parsing bug)
Definition: types.h:39
@ JO_KEYBOARD_F2
Definition: keyboard.h:89
@ JO_KEYBOARD_UP
Definition: keyboard.h:80
@ JO_KEYBOARD_BACKSPACE
Definition: keyboard.h:83
@ JO_KEYBOARD_F1
Definition: keyboard.h:88
@ JO_KEYBOARD_F3
Definition: keyboard.h:90
@ JO_KEYBOARD_F8
Definition: keyboard.h:95
@ JO_KEYBOARD_DOWN
Definition: keyboard.h:79
@ JO_KEYBOARD_F12
Definition: keyboard.h:99
jo_keyboard_special_key
Keyboard special keys.
Definition: keyboard.h:75
@ JO_KEYBOARD_F4
Definition: keyboard.h:91
@ JO_KEYBOARD_ENTER
Definition: keyboard.h:82
char __internal_keyboard_normal_mapping[]
Keyboard mapping (internal engine usage)
@ JO_KEYBOARD_F7
Definition: keyboard.h:94
static __jo_force_inline unsigned char jo_keyboard_get_char(void)
Definition: keyboard.h:104
@ JO_KEYBOARD_END
Definition: keyboard.h:87
@ JO_KEYBOARD_F10
Definition: keyboard.h:97
@ JO_KEYBOARD_NO_SPECIAL_KEY
Definition: keyboard.h:76
@ JO_KEYBOARD_ESCAPE
Definition: keyboard.h:81
jo_keyboard_special_key jo_keyboard_get_special_key(void)