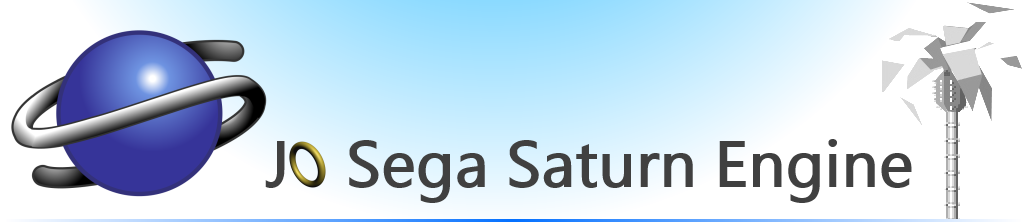 |
Jo Engine
2024.04.28
Jo Sega Saturn Engine
|
Go to the documentation of this file.
41 # define JO_MAP_NO_COLLISION (-2147483647)
71 #ifdef JO_COMPILE_WITH_FS_SUPPORT
87 bool jo_map_load_from_file(
const unsigned int layer,
const short depth,
const char *
const sub_dir,
const char *
const filename);
97 bool jo_map_create(
const unsigned int layer,
const unsigned short max_tile_count,
const short depth);
107 #
if JO_MAX_SPRITE > 255
108 const unsigned short sprite_id,
110 const unsigned char sprite_id,
112 const unsigned char attribute);
121 void jo_map_add_animated_tile(
const unsigned int layer,
const short x,
const short y,
const unsigned char anim_id,
const unsigned char attribute);
133 void jo_map_draw(
const unsigned int layer,
const short screen_x,
const short screen_y);
151 #ifdef JO_COMPILE_WITH_3D_SUPPORT
152 void jo_map_3d_draw(
const unsigned int layer,
const int x,
const int y,
const int z);
153 #endif // JO_COMPILE_WITH_3D_SUPPORT
void jo_map_move_tiles_by_attribute(const unsigned int layer, const unsigned char attribute_filter, const short incr_x, const short incr_y)
Move specific tiles by their attributes.
bool jo_map_load_from_file(const unsigned int layer, const short depth, const char *const sub_dir, const char *const filename)
Load a sprite map on a specific layer using MAP file format.
bool jo_map_create(const unsigned int layer, const unsigned short max_tile_count, const short depth)
Create a new sprite map.
void jo_map_3d_draw(const unsigned int layer, const int x, const int y, const int z)
void jo_map_draw(const unsigned int layer, const short screen_x, const short screen_y)
Display the specific layer.
void jo_map_free(const unsigned int layer)
Destroy the specific layer.
int jo_map_hitbox_detection_custom_boundaries(const unsigned int layer, const int x, const int y, const int w, const int h)
Fast method to get if a square intersects with the map (HitBox processing) based on screen coord.
void jo_map_add_animated_tile(const unsigned int layer, const short x, const short y, const unsigned char anim_id, const unsigned char attribute)
Add an animated tile on the specific layer.
int jo_map_per_pixel_vertical_collision(const unsigned int layer, int x, int y, unsigned char *attribute)
Method to get vertical distance between our point (x, y) and the surface of the map (example: hill)
void jo_map_add_tile(const unsigned int layer, const short x, const short y, const unsigned char sprite_id, const unsigned char attribute)
Add a tile on the specific layer.
void jo_map_draw_background(const unsigned int layer, const short x, const short y)
Draw the specific layer on the background (NBG1)