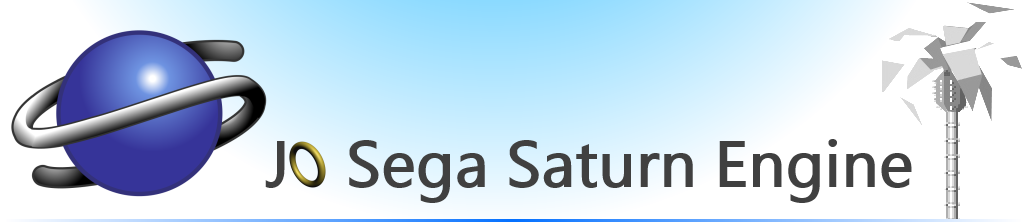 |
Jo Engine
2024.04.28
Jo Sega Saturn Engine
|
Go to the documentation of this file.
36 # define __JO_MATH_H__
48 # define JO_PI (3.1415927)
51 # define JO_PI_2 (1.5707963)
66 # define JO_MULT_BY_2(X) ((X) << 1)
72 # define JO_MULT_BY_4(X) ((X) << 2)
78 # define JO_MULT_BY_8(X) ((X) << 3)
84 # define JO_MULT_BY_16(X) ((X) << 4)
90 # define JO_MULT_BY_32(X) ((X) << 5)
96 # define JO_MULT_BY_64(X) ((X) << 6)
102 # define JO_MULT_BY_128(X) ((X) << 7)
108 # define JO_MULT_BY_256(X) ((X) << 8)
114 # define JO_MULT_BY_1024(X) ((X) << 10)
120 # define JO_MULT_BY_2048(X) ((X) << 11)
126 # define JO_MULT_BY_4096(X) ((X) << 12)
132 # define JO_MULT_BY_32768(X) ((X) << 15)
138 # define JO_MULT_BY_65536(X) ((X) << 16)
144 # define JO_DIV_BY_2(X) ((X) >> 1)
150 # define JO_DIV_BY_4(X) ((X) >> 2)
156 # define JO_DIV_BY_8(X) ((X) >> 3)
162 # define JO_DIV_BY_16(X) ((X) >> 4)
168 # define JO_DIV_BY_32(X) ((X) >> 5)
174 # define JO_DIV_BY_64(X) ((X) >> 6)
180 # define JO_DIV_BY_1024(X) ((X) >> 10)
186 # define JO_DIV_BY_32768(X) ((X) >> 15)
192 # define JO_DIV_BY_65536(X) ((X) >> 16)
198 # define JO_DIV_BY_2147483648(X) ((X) >> 31)
213 # define JO_ZERO(X) X ^= X
220 # define JO_MOD_POW2(N, M) ((N) & ((M) - 1))
225 # define JO_ABS(X) ((X) < 0 ? -(X) : (X))
230 # define JO_FABS(X) ((X) < 0.0f ? -(X) : (X))
236 # define JO_MIN(A, B) (((A) < (B)) ? (A) : (B))
242 # define JO_MAX(A, B) (((A) > (B)) ? (A) : (B))
247 # define JO_CHANGE_SIGN(X) (-(X))
250 # define JO_FLOAT_EPSILON (0.00001f)
256 # define JO_FLOAT_NEARLY_EQUALS(A, B) ((A) <= ((B) + JO_FLOAT_EPSILON) && (A) >= ((B) - JO_FLOAT_EPSILON))
261 # define JO_IS_FLOAT_NULL(A) ((A) <= JO_FLOAT_EPSILON && (A) >= (-JO_FLOAT_EPSILON))
266 # define JO_RAD_TO_DEG(A) (180.0 * (A) / JO_PI)
271 # define JO_DEG_TO_RAD(A) (JO_PI * (A) / 180.0)
276 # define JO_IS_ODD(A) ((A) & 1)
282 # define JO_SWAP(A, B) { (A) = (A) ^ (B); (B) = (A) ^ (B); (A) = (A) ^ (B); }
288 # define JO_PERCENT_USED(TOTAL, FREE) (int)(100.0f / (float)(TOTAL) * (float)((TOTAL) - (FREE)))
293 # define JO_SQUARE(A) ((A) * (A))
298 # define JO_BCD_INT(BCD) (((BCD & 0xF0) >> 4) * 10 + (BCD & 0x0F))
313 # define JO_SET_FLAGS(BYTEFIELD, FLAGS) BYTEFIELD = (FLAGS)
318 # define JO_SET_ALL_FLAGS(BYTEFIELD) BYTEFIELD = (~0)
324 # define JO_ADD_FLAG(BYTEFIELD, FLAG) BYTEFIELD |= (FLAG)
330 # define JO_REMOVE_FLAG(BYTEFIELD, FLAG) BYTEFIELD &= ~(FLAG)
335 # define JO_REMOVE_ALL_FLAGS(BYTEFIELD) BYTEFIELD = 0
341 # define JO_HAS_FLAG(BYTEFIELD, FLAG) ((BYTEFIELD & FLAG) == FLAG)
357 # define JO_FIXED_0 (0)
359 # define JO_FIXED_1_DIV_2 (32768)
361 # define JO_FIXED_1 (65536)
363 # define JO_FIXED_2 JO_INT_TO_FIXED(2)
365 # define JO_FIXED_4 JO_INT_TO_FIXED(4)
367 # define JO_FIXED_8 JO_INT_TO_FIXED(8)
369 # define JO_FIXED_16 JO_INT_TO_FIXED(16)
371 # define JO_FIXED_32 JO_INT_TO_FIXED(32)
373 # define JO_FIXED_120 JO_INT_TO_FIXED(120)
375 # define JO_FIXED_150 JO_INT_TO_FIXED(150)
377 # define JO_FIXED_180 JO_INT_TO_FIXED(180)
379 # define JO_FIXED_360 JO_INT_TO_FIXED(360)
382 # define JO_FIXED_MIN (-2147483647)
384 # define JO_FIXED_MAX (2147483647)
386 # define JO_FIXED_EPSILON (1)
388 # define JO_FIXED_OVERFLOW (0x80000000)
390 # define JO_FIXED_PI (205887)
392 # define JO_FIXED_PI_2 (411775)
394 # define JO_FIXED_180_DIV_PI (3754936)
396 # define JO_FIXED_PI_DIV_180 (1144)
398 # define JO_FIXED_PI_DIV_2 (102944)
400 # define JO_FIXED_1_DIV (1.0f / 65536.0f)
405 # define JO_INT_TO_FIXED(X) JO_MULT_BY_65536(X)
409 # define JO_FIXED_TO_INT(X) JO_DIV_BY_65536(X)
523 return (x & 0xFFFF0000UL) + (x & 0x0000FFFFUL ?
JO_FIXED_1 : 0);
532 return (x & 0xFFFF0000ul);
561 return (
jo_random(max) / multiple) * multiple;
592 unsigned int i = *(
unsigned int*)(
void *)&value;
595 return *(
float*)(
void *)&i;
631 # define __JO_DEG_TO_ANGLE_MAGIC (182)
639 #ifdef JO_COMPILE_WITH_FAST_BUT_LESS_ACCURATE_MATH
642 return ((ANGLE)((65536.0 * (deg)) / 360.0));
652 #ifdef JO_COMPILE_WITH_FAST_BUT_LESS_ACCURATE_MATH
659 #if JO_COMPILE_USING_SGL
894 return (3.14159f - 1.57079f * angle);
962 result->x = a->x + b->x;
963 result->y = a->y + b->y;
964 result->z = a->z + b->z;
988 result->x = a->x + b->x;
989 result->y = a->y + b->y;
990 result->z = a->z + b->z;
1002 result->x = a->x - b->x;
1003 result->y = a->y - b->y;
1004 result->z = a->z - b->z;
1071 result->
x = a->
x + b->
x;
1072 result->
y = a->
y + b->
y;
1073 result->
z = a->
z + b->
z;
1084 result->
x = a->
x + s;
1085 result->
y = a->
y + s;
1086 result->
z = a->
z + s;
1097 result->
x = a->
x - b->
x;
1098 result->
y = a->
y - b->
y;
1099 result->
z = a->
z - b->
z;
1110 result->
x = a->
x - s;
1111 result->
y = a->
y - s;
1112 result->
z = a->
z - s;
1123 result->
x = a->
x * b->
x;
1124 result->
y = a->
y * b->
y;
1125 result->
z = a->
z * b->
z;
1136 result->
x = a->
x * s;
1137 result->
y = a->
y * s;
1138 result->
z = a->
z * s;
1149 result->
x = a->
x / b->
x;
1150 result->
y = a->
y / b->
y;
1151 result->
z = a->
z / b->
z;
1162 result->
x = a->
x / s;
1163 result->
y = a->
y / s;
1164 result->
z = a->
z / s;
1183 return (a->
x * b->
x + a->
y * b->
y + a->
z * b->
z);
1196 result->
x = a->
x / len;
1197 result->
y = a->
y / len;
1198 result->
z = a->
z / len;
1226 result->
x = a->
y * b->
z - a->
z * b->
y;
1227 result->
y = a->
z * b->
x - a->
x * b->
z;
1228 result->
z = a->
x * b->
y - a->
y * b->
x;
1258 for (i = 1; i < 15; ++i)
1273 for (i = 1; i < 15; ++i)
1274 result->
table[i] = 0;
1288 result->m30 = offset->x;
1289 result->m31 = offset->y;
1290 result->m32 = offset->z;
1300 result->m30 = offset->
x;
1301 result->m31 = offset->
y;
1302 result->m32 = offset->
z;
1312 result->m00 = scale->x;
1313 result->m11 = scale->y;
1314 result->m22 = scale->z;
1324 result->m00 = scale->
x;
1325 result->m11 = scale->
y;
1326 result->m22 = scale->
z;
1338 result->m21 = -result->m12;
1339 result->m22 = result->m11;
1351 result->m21 = -result->m12;
1352 result->m22 = result->m11;
1364 result->m02 = -result->m20;
1365 result->m22 = result->m00;
1377 result->m02 = -result->m20;
1378 result->m22 = result->m00;
1390 result->m10 = -result->m01;
1391 result->m11 = result->m00;
1403 result->m10 = -result->m01;
1404 result->m11 = result->m00;
1414 result->m00 = matrix->m00;
1415 result->m10 = matrix->m01;
1416 result->m20 = matrix->m02;
1417 result->m30 = matrix->m03;
1418 result->m01 = matrix->m10;
1419 result->m11 = matrix->m11;
1420 result->m21 = matrix->m12;
1421 result->m31 = matrix->m13;
1422 result->m02 = matrix->m20;
1423 result->m12 = matrix->m21;
1424 result->m22 = matrix->m22;
1425 result->m32 = matrix->m23;
1426 result->m03 = matrix->m30;
1427 result->m13 = matrix->m31;
1428 result->m23 = matrix->m32;
1429 result->m33 = matrix->m33;
1439 result->m00 = matrix->m00;
1440 result->m10 = matrix->m01;
1441 result->m20 = matrix->m02;
1442 result->m30 = matrix->m03;
1443 result->m01 = matrix->m10;
1444 result->m11 = matrix->m11;
1445 result->m21 = matrix->m12;
1446 result->m31 = matrix->m13;
1447 result->m02 = matrix->m20;
1448 result->m12 = matrix->m21;
1449 result->m22 = matrix->m22;
1450 result->m32 = matrix->m23;
1451 result->m03 = matrix->m30;
1452 result->m13 = matrix->m31;
1453 result->m23 = matrix->m32;
1454 result->m33 = matrix->m33;
1484 for (i = 0; i < 4; ++i)
1486 for (j = 0; j < 4; ++j)
1489 for (k = 0; k < 4; ++k)
1491 result->
m[i][j] = sum;
1509 for (i = 0; i < 4; ++i)
1511 for (j = 0; j < 4; ++j)
1514 for (k = 0; k < 4; ++k)
1515 sum += a->
m[k][j] * b->
m[i][k];
1516 result->
m[i][j] = sum;
1535 result->m00 = c + normalized_axis.
x * normalized_axis.
x * a;
1536 result->m10 = normalized_axis.
x * normalized_axis.
y * a - normalized_axis.
z * s;
1537 result->m20 = normalized_axis.
x * normalized_axis.
z * a + normalized_axis.
y * s;
1538 result->m01 = normalized_axis.
y * normalized_axis.
x * a + normalized_axis.
z * s;
1539 result->m11 = c + normalized_axis.
y * normalized_axis.
y * a;
1540 result->m21 = normalized_axis.
y * normalized_axis.
z * a - normalized_axis.
x * s;
1541 result->m02 = normalized_axis.
z * normalized_axis.
x * a - normalized_axis.
y * s;
1542 result->m12 = normalized_axis.
z * normalized_axis.
y * a + normalized_axis.
x * s;
1543 result->m22 = c + normalized_axis.
z * normalized_axis.
z * a;
1556 const float back,
const float front,
jo_matrixf *
const result)
1559 result->m00 = 2 / (right - left);
1560 result->m11 = 2 / (top - bottom);
1561 result->m22 = 2 / (back - front);
1562 result->m30 = -(right + left) / (right - left);
1563 result->m31 = -(top + bottom) / (top - bottom);
1564 result->m32 = -(back + front) / (back - front);
1576 const float near_view_distance,
const float far_view_distance,
jo_matrixf *
const result)
1579 result->m11 = 1.0f /
jo_tan_radf((vertical_field_of_view_in_deg / 180 *
JO_PI) / 2.0f);
1580 result->m00 = result->m11 / aspect_ratio;
1581 result->m22 = (far_view_distance + near_view_distance) / (near_view_distance - far_view_distance);
1582 result->m32 = (2 * far_view_distance * near_view_distance) / (near_view_distance - far_view_distance);
1629 float c00 = matrix->m11 * matrix->m22 - matrix->m12 * matrix->m21;
1630 float c10 = -(matrix->m01 * matrix->m22 - matrix->m02 * matrix->m21);
1631 float c20 = matrix->m01 * matrix->m12 - matrix->m02 * matrix->m11;
1632 float det = matrix->m00 * c00 + matrix->m10 * c10 + matrix->m20 * c20;
1636 float c01 = -(matrix->m10 * matrix->m22 - matrix->m12 * matrix->m20);
1637 float c11 = matrix->m00 * matrix->m22 - matrix->m02 * matrix->m20;
1638 float c21 = -(matrix->m00 * matrix->m12 - matrix->m02 * matrix->m10);
1639 float c02 = matrix->m10 * matrix->m21 - matrix->m11 * matrix->m20;
1640 float c12 = -(matrix->m00 * matrix->m21 - matrix->m01 * matrix->m20);
1641 float c22 = matrix->m00 * matrix->m11 - matrix->m01 * matrix->m10;
1642 float i00 = c00 / det;
1643 float i10 = c01 / det;
1644 float i20 = c02 / det;
1645 float i01 = c10 / det;
1646 float i11 = c11 / det;
1647 float i21 = c12 / det;
1648 float i02 = c20 / det;
1649 float i12 = c21 / det;
1650 float i22 = c22 / det;
1655 result->m30 = -(i00 * matrix->m30 + i10 * matrix->m31 + i20 * matrix->m32);
1659 result->m31 = -(i01 * matrix->m30 + i11 * matrix->m31 + i21 * matrix->m32);
1663 result->m32 = -(i02 * matrix->m30 + i12 * matrix->m31 + i22 * matrix->m32);
1674 float w = matrix->m03 * position->
x + matrix->m13 * position->
y + matrix->m23 * position->
z + matrix->m33;
1675 result->
x = matrix->m00 * position->
x + matrix->m10 * position->
y + matrix->m20 * position->
z + matrix->m30;
1676 result->
y = matrix->m01 * position->
x + matrix->m11 * position->
y + matrix->m21 * position->
z + matrix->m31;
1677 result->
z = matrix->m02 * position->
x + matrix->m12 * position->
y + matrix->m22 * position->
z + matrix->m32;
1678 if (w != 0 && w != 1)
1694 float w = matrix->m03 * direction->
x + matrix->m13 * direction->
y + matrix->m23 * direction->
z;
1695 result->
x = matrix->m00 * direction->
x + matrix->m10 * direction->
y + matrix->m20 * direction->
z;
1696 result->
y = matrix->m01 * direction->
x + matrix->m11 * direction->
y + matrix->m21 * direction->
z;
1697 result->
z = matrix->m02 * direction->
x + matrix->m12 * direction->
y + matrix->m22 * direction->
z;
1698 if (w != 0 && w != 1)
1733 return (
JO_ABS(f) < 0.00000001f);
1748 const int x2,
const int y2,
const int w2,
const int h2)
1750 return ((x1 < x2 + w2) && (x2 < x1 + w1)) && ((y1 < y2 + h2) && (y2 < y1 + h1));
static __jo_force_inline void jo_matrixf_mul(const jo_matrixf *const a, const jo_matrixf *const b, jo_matrixf *const result)
Multiply 2 matrix (using floating numbers)
Definition: math.h:1502
static __jo_force_inline int jo_sin_mult(const int value, const int deg)
Fast sinus multiplication.
Definition: math.h:740
static __jo_force_inline float jo_sin_radf(const float rad)
Sinus computation.
Definition: math.h:730
float jo_rsqrt(float value)
Fast Reciprocal Square root using floating number.
static __jo_force_inline float jo_cosf_mult(const float value, const int deg)
Fast cosinus multiplication.
Definition: math.h:825
int jo_gcd(int a, int b)
Get the greatest common divisor.
@ RIGHT
Definition: types.h:305
#define JO_FIXED_360
Fixed floating point value for 360.
Definition: math.h:379
static __jo_force_inline jo_fixed jo_fixed_deg2rad(const jo_fixed deg)
Convert fixed degree to fixed radian.
Definition: math.h:503
static __jo_force_inline void jo_matrix_rotation_y_rad(const float angle_in_rad, jo_matrix *const result)
Creates rotating matrix (Y axis) (using fixed numbers)
Definition: math.h:1359
static __jo_force_inline void jo_vector4_fixed_add(const jo_vector4_fixed *const a, const jo_vector4_fixed *const b, jo_vector4_fixed *const result)
Add 2 vectors4 (using fixed numbers)
Definition: math.h:986
@ UP_RIGHT
Definition: types.h:309
float y
Definition: types.h:134
#define JO_RAD_TO_DEG(A)
Convert radians to degrees.
Definition: math.h:266
static __jo_force_inline void jo_vectorf_subs(const jo_vectorf *const a, const float s, jo_vectorf *const result)
Subtract value to vector (using floating numbers)
Definition: math.h:1108
#define JO_FIXED_180
Fixed floating point value for 180.
Definition: math.h:377
int jo_random(int max)
Get a random number.
static __jo_force_inline float jo_vectorf_angle_between_radf(const jo_vectorf *const a, const jo_vectorf *const b)
Get the angle between 2 vectors (using floating numbers)
Definition: math.h:1236
static __jo_force_inline void jo_matrix_rotation_z_rad(const float angle_in_rad, jo_matrix *const result)
Creates rotating matrix (Z axis) (using fixed numbers)
Definition: math.h:1385
static __jo_force_inline void jo_matrixf_scaling(const jo_vectorf *const scale, jo_matrixf *const result)
Creates scaling matrix (using floating numbers)
Definition: math.h:1321
@ UP_LEFT
Definition: types.h:308
static __jo_force_inline ANGLE jo_DEGtoANG(const float deg)
Replacement for DEGtoANG using floating number.
Definition: math.h:637
static __jo_force_inline jo_fixed jo_fixed_floor(const jo_fixed x)
Returns the largest (fixed) integer value less than or equal to x.
Definition: math.h:530
float z
Definition: types.h:135
static __jo_force_inline jo_fixed jo_sin(const int deg)
Fast sinus computation.
Definition: math.h:700
#define JO_INT_TO_FIXED(X)
Convert int to jo_fixed.
Definition: math.h:405
static __jo_force_inline jo_fixed jo_sin_rad(const float rad)
Sinus computation.
Definition: math.h:720
static __jo_force_inline float jo_cos_radf(const float rad)
Cosinus computation.
Definition: math.h:805
@ DOWN_RIGHT
Definition: types.h:311
static __jo_force_inline void jo_vector_fixed_add(const jo_vector_fixed *const a, const jo_vector_fixed *const b, jo_vector_fixed *const result)
Add 2 vectors (using fixed numbers)
Definition: math.h:960
static __jo_force_inline void jo_matrixf_transpose(const jo_matrixf *const matrix, jo_matrixf *const result)
Creates transpose matrix (using floating numbers)
Definition: math.h:1437
static __jo_force_inline void jo_vector4_swap(jo_vector4_fixed *const a, jo_vector4_fixed *const b)
Dot product of 2 vectors4 (using fixed numbers)
Definition: math.h:1036
static __jo_force_inline void jo_vector4_fixed_cross(const jo_vector4_fixed *const a, const jo_vector4_fixed *const b, jo_vector4_fixed *const result)
Cross product of 2 vectors4 (using fixed numbers)
Definition: math.h:1013
static __jo_force_inline void jo_vectorf_cross(const jo_vectorf *const a, const jo_vectorf *const b, jo_vectorf *const result)
Cross 2 vectors (using floating numbers)
Definition: math.h:1224
static __jo_force_inline float jo_cosf(const int deg)
Cosinus computation.
Definition: math.h:785
static __jo_force_inline void jo_vectorf_adds(const jo_vectorf *const a, const float s, jo_vectorf *const result)
Add value to vector (using floating numbers)
Definition: math.h:1082
Vector4 for 3D computation using fixed number.
Definition: types.h:165
static __jo_force_inline float jo_acos_radf(const float angle)
Fast Arc Cosinus computation.
Definition: math.h:892
static __jo_force_inline void jo_matrixf_identity(jo_matrixf *const result)
Creates the identity matrix (using floating numbers)
Definition: math.h:1269
static __jo_force_inline void jo_vectorf_sub(const jo_vectorf *const a, const jo_vectorf *const b, jo_vectorf *const result)
Subtract 2 vectors (using floating numbers)
Definition: math.h:1095
@ UP
Definition: types.h:306
static __jo_force_inline ANGLE jo_DEGtoANG_int(const int deg)
Replacement for DEGtoANG using interger.
Definition: math.h:650
#define JO_MULT_BY_65536(X)
Multiply a variable by 65536.
Definition: math.h:138
static __jo_force_inline float jo_sqrtf(float value)
Fast Square root using floating number.
Definition: math.h:590
static __jo_force_inline void jo_matrix_mul(const jo_matrix *const a, const jo_matrix *const b, jo_matrix *const result)
Multiply 2 matrix (using fixed numbers)
Definition: math.h:1477
static __jo_force_inline ANGLE jo_fixed_deg2ANGLE(const jo_fixed deg)
Convert fixed degree to SGL ANGLE.
Definition: math.h:676
#define __JO_DEG_TO_ANGLE_MAGIC
See https://github.com/johannes-fetz/joengine/issues/42.
Definition: math.h:631
#define JO_FIXED_TO_INT(X)
Convert jo_fixed to int.
Definition: math.h:409
static __jo_force_inline bool jo_square_intersect(const int x1, const int y1, const int w1, const int h1, const int x2, const int y2, const int w2, const int h2)
Fast method to get if two square intersects (HitBox processing)
Definition: math.h:1747
void jo_vector_fixed_compute_bezier_point(const jo_fixed t, jo_vector_fixed p0, jo_vector_fixed p1, jo_vector_fixed p2, jo_vector_fixed p3, jo_vector_fixed *result)
Compute cubic bezier curve point for vectors (using fixed numbers)
static __jo_force_inline jo_fixed jo_fixed_ceil(const jo_fixed x)
Returns the smallest (fixed) integer value greater than or equal to x.
Definition: math.h:521
static __jo_force_inline ANGLE jo_fixed_rad2ANGLE(const jo_fixed rad)
Convert fixed radian to SGL ANGLE.
Definition: math.h:665
jo_fixed m[4][4]
Definition: types.h:187
static __jo_force_inline bool jo_is_float_equals_zero(const float f)
Check if float almost equals 0.
Definition: math.h:1731
static __jo_force_inline void jo_matrixf_rotation(const float angle_in_rad, const jo_vectorf *const axis, jo_matrixf *const result)
Creates a matrix to rotate around an axis by a given angle in radiant (using floating numbers)
Definition: math.h:1526
jo_fixed jo_fixed_cos(jo_fixed rad)
Fast cosinus computation using fixed number.
float table[16]
Definition: types.h:174
static __jo_force_inline jo_fixed jo_cos(const int deg)
Fast cosinus computation.
Definition: math.h:775
static __jo_force_inline short jo_direction_to_angle(const jo_8_directions direction)
Convert jo_8_directions to angle in degree.
Definition: math.h:1774
static __jo_force_inline void jo_matrixf_translation(const jo_vectorf *const offset, jo_matrixf *const result)
Creates translation matrix (using floating numbers)
Definition: math.h:1297
static __jo_force_inline void jo_matrixf_mul_dir(const jo_matrixf *const matrix, const jo_vectorf *const direction, jo_vectorf *const result)
Multiplies a 4x4 matrix with a 3D vector representing a direction in 3D space (using floating numbers...
Definition: math.h:1691
jo_fixed table[16]
Definition: types.h:188
static __jo_force_inline jo_fixed jo_fixed_wrap_to_180(jo_fixed deg)
Wrap deg in [-180 180].
Definition: math.h:462
static __jo_force_inline void jo_matrix_transpose(const jo_matrix *const matrix, jo_matrix *const result)
Creates transpose matrix (using fixed numbers)
Definition: math.h:1412
static __jo_force_inline void jo_vectorf_mul(const jo_vectorf *const a, const jo_vectorf *const b, jo_vectorf *const result)
Multiply 2 vectors (using floating numbers)
Definition: math.h:1121
#define JO_ZERO(X)
Set a variable to zero.
Definition: math.h:213
static __jo_force_inline void jo_matrix_scaling(const jo_vector_fixed *const scale, jo_matrix *const result)
Creates scaling matrix (using fixed numbers)
Definition: math.h:1309
static __jo_force_inline float jo_fixed2float(const jo_fixed x)
Convert jo engine fixed to float.
Definition: math.h:442
jo_fixed jo_fixed_dot(jo_fixed ptA[3], jo_fixed ptB[3])
Dot product two fixed 3D points.
#define JO_ABS(X)
Get the absolute value of X.
Definition: math.h:225
static __jo_force_inline void jo_matrixf_rotation_x_rad(const float angle_in_rad, jo_matrixf *const result)
Creates rotating matrix (X axis) (using floating numbers)
Definition: math.h:1346
static __jo_force_inline int jo_atan2f(const float y, const float x)
Fast ATAN2 computation in degree.
Definition: math.h:921
float jo_atan2f_rad(const float y, const float x)
Fast ATAN2 computation in radian.
static __jo_force_inline void jo_matrixf_look_at(const jo_vectorf *const from, const jo_vectorf *const to, const jo_vectorf *const up, jo_matrixf *const result)
Builds a transformation matrix for a camera (using floating numbers)
Definition: math.h:1593
static __jo_force_inline void jo_vectorf_normalize(const jo_vectorf *const a, jo_vectorf *const result)
Normalize a vector (using floating numbers)
Definition: math.h:1191
static __jo_force_inline void jo_matrix_rotation_x_rad(const float angle_in_rad, jo_matrix *const result)
Creates rotating matrix (X axis) (using fixed numbers)
Definition: math.h:1333
#define __jo_force_inline
force inline attribute (and prevent Doxygen prototype parsing bug)
Definition: types.h:39
static __jo_force_inline float jo_tan_radf(const float rad)
Tangent computation.
Definition: math.h:874
static __jo_force_inline void jo_vectorf_add(const jo_vectorf *const a, const jo_vectorf *const b, jo_vectorf *const result)
Add 2 vectors (using floating numbers)
Definition: math.h:1069
static __jo_force_inline jo_fixed jo_tan(const int deg)
Fast tangent computation.
Definition: math.h:844
int jo_fixed
Fixed point Q16.16 number.
Definition: types.h:49
#define JO_FLOAT_EPSILON
Float minimum positive value.
Definition: math.h:250
static __jo_force_inline void jo_matrixf_invert_affine(const jo_matrixf *const matrix, jo_matrixf *const result)
Inverts an affine transformation matrix (using floating numbers)
Definition: math.h:1627
#define JO_FIXED_PI_2
Fixed value of 2 PI.
Definition: math.h:392
int jo_random_seed
Get or set current random seed.
static __jo_force_inline jo_fixed jo_int2fixed(const int x)
Convert int to jo engine fixed.
Definition: math.h:415
4x4 MATRIX for 3D computation using fixed number
Definition: types.h:186
#define JO_FIXED_PI
Fixed value of PI.
Definition: math.h:390
unsigned int jo_sqrt(unsigned int value)
Fast square root.
void jo_planar_rotate(const jo_pos2D *const point, const jo_pos2D *const origin, const int angle, jo_pos2D *const result)
Rotate a point on the plan with a specific origin.
Vector for 3D computation using fixed number.
Definition: types.h:150
static __jo_force_inline int jo_fixed2int(const jo_fixed x)
Convert jo engine fixed to int.
Definition: math.h:424
float x
Definition: types.h:133
#define JO_FIXED_PI_DIV_180
Fixed value of PI/180.
Definition: math.h:396
static __jo_force_inline void jo_matrix_identity(jo_matrix *const result)
Creates the identity matrix (using fixed numbers)
Definition: math.h:1254
jo_fixed w
Definition: types.h:167
static __jo_force_inline float jo_vectorf_length(const jo_vectorf *const a)
Get the length of a vector (using floating numbers)
Definition: math.h:1171
static __jo_force_inline void jo_vectorf_div(const jo_vectorf *const a, const jo_vectorf *const b, jo_vectorf *const result)
Divide 2 vectors (using floating numbers)
Definition: math.h:1147
static __jo_force_inline void jo_vectorf_divs(const jo_vectorf *const a, const float s, jo_vectorf *const result)
Divide value to vector (using floating numbers)
Definition: math.h:1160
static __jo_force_inline int jo_cos_mult(const int value, const int deg)
Fast cosinus multiplication.
Definition: math.h:815
static __jo_force_inline float jo_tanf(const float deg)
Tangent computation.
Definition: math.h:854
#define JO_SWAP(A, B)
Swap A and B values.
Definition: math.h:282
void jo_vectorf_compute_bezier_point(const float t, jo_vectorf p0, jo_vectorf p1, jo_vectorf p2, jo_vectorf p3, jo_vectorf *result)
Compute cubic bezier curve point for vectors (using floating numbers)
static __jo_force_inline jo_fixed jo_lerp(const jo_fixed v0, const jo_fixed v1, const jo_fixed t)
Linear interpolation which guarantees v = v1 when t = 1.
Definition: math.h:1722
static __jo_force_inline float jo_sinf_mult(const float value, const int deg)
Fast sinus multiplication.
Definition: math.h:750
@ DOWN
Definition: types.h:307
static __jo_force_inline void jo_vector4_fixed_sub(const jo_vector4_fixed *const a, const jo_vector4_fixed *const b, jo_vector4_fixed *const result)
Substract 2 vectors4 (using fixed numbers)
Definition: math.h:1000
static __jo_force_inline void jo_matrixf_rotation_y_rad(const float angle_in_rad, jo_matrixf *const result)
Creates rotating matrix (Y axis) (using floating numbers)
Definition: math.h:1372
#define JO_PI
PI value.
Definition: math.h:48
static __jo_force_inline int jo_random_using_multiple(int max, int multiple)
Get a random number with a specific multiple.
Definition: math.h:559
static __jo_force_inline void jo_matrix_translation(const jo_vector_fixed *const offset, jo_matrix *const result)
Creates translation matrix (using fixed numbers)
Definition: math.h:1285
static __jo_force_inline jo_fixed jo_fixed_rad2deg(const jo_fixed rad)
Convert fixed radian to fixed degree.
Definition: math.h:512
#define JO_SQUARE(A)
Square computation (x²)
Definition: math.h:293
4x4 MATRIX for 3D computation using floating numbers
Definition: types.h:172
float m[4][4]
Definition: types.h:173
static __jo_force_inline float jo_sinf(const int deg)
Sinus computation.
Definition: math.h:710
static __jo_force_inline jo_fixed jo_cos_rad(const float rad)
Cosinus computation.
Definition: math.h:795
static __jo_force_inline float jo_vectorf_dot(const jo_vectorf *const a, const jo_vectorf *const b)
Get the dot product of 2 vectors (using floating numbers)
Definition: math.h:1181
jo_8_directions
8 directions definition
Definition: types.h:302
static __jo_force_inline jo_fixed jo_fixed_wrap_to_pi(jo_fixed rad)
Wrap rad in [−pi pi].
Definition: math.h:451
jo_fixed jo_fixed_sqrt(jo_fixed value)
Fast Square root using fixed number.
2D position
Definition: types.h:53
static __jo_force_inline void jo_matrix_mul_vector4(const jo_matrix *const m, const jo_vector4_fixed *const v, jo_vector4_fixed *const result)
Multiply a matrix by a vector4 (using fixed numbers)
Definition: math.h:1463
jo_fixed jo_fixed_mult(jo_fixed x, jo_fixed y)
Multiply to fixed number.
@ DOWN_LEFT
Definition: types.h:310
jo_fixed jo_fixed_sin(jo_fixed rad)
Fast sinus computation using fixed number.
static __jo_force_inline void jo_matrixf_mul_pos(const jo_matrixf *const matrix, const jo_vectorf *const position, jo_vectorf *const result)
Multiplies a 4x4 matrix with a 3D vector representing a point in 3D space (using floating numbers)
Definition: math.h:1671
#define JO_FIXED_1_DIV
Fixed value of 1/65536.
Definition: math.h:400
#define JO_FIXED_1
Fixed floating point value for 1.
Definition: math.h:361
static __jo_force_inline jo_fixed jo_float2fixed(const float x)
Convert float to jo engine fixed (avoid usage of GCC Soft Float)
Definition: math.h:433
#define JO_FABS(X)
Get the absolute value of X.
Definition: math.h:230
static __jo_force_inline void jo_vectorf_proj(const jo_vectorf *const v, const jo_vectorf *const onto, jo_vectorf *const result)
Compute projection vector (using floating numbers)
Definition: math.h:1213
jo_fixed jo_fixed_rsqrt(jo_fixed value)
Fast Reciprocal Square root using fixed number.
jo_fixed jo_fixed_pow(jo_fixed x, jo_fixed y)
x raised to the power of the integer part of y
Vector for 3D computation using floating numbers.
Definition: types.h:132
static __jo_force_inline jo_fixed jo_tan_rad(const float rad)
Tangent computation.
Definition: math.h:864
static __jo_force_inline jo_fixed jo_vector4_fixed_dot(const jo_vector4_fixed *const a, const jo_vector4_fixed *const b)
Dot product of 2 vectors4 (using fixed numbers)
Definition: math.h:1026
static __jo_force_inline void jo_matrixf_perspective(const float vertical_field_of_view_in_deg, const float aspect_ratio, const float near_view_distance, const float far_view_distance, jo_matrixf *const result)
Creates a perspective projection matrix for a camera (using floating numbers)
Definition: math.h:1575
#define JO_FIXED_180_DIV_PI
Fixed value of 180/PI.
Definition: math.h:394
@ LEFT
Definition: types.h:304
static __jo_force_inline void jo_vectorf_muls(const jo_vectorf *const a, const float s, jo_vectorf *const result)
Multiply value to vector (using floating numbers)
Definition: math.h:1134
static __jo_force_inline void jo_matrixf_ortho(const float left, const float right, const float bottom, const float top, const float back, const float front, jo_matrixf *const result)
Creates an orthographic projection matrix (using floating numbers)
Definition: math.h:1555
jo_fixed jo_fixed_div(jo_fixed dividend, jo_fixed divisor)
Divide fixed-point numbers (expresses dividend / divisor)
static __jo_force_inline void jo_matrixf_rotation_z_rad(const float angle_in_rad, jo_matrixf *const result)
Creates rotating matrix (Z axis) (using floating numbers)
Definition: math.h:1398
static __jo_force_inline void jo_vector_fixed_muls(const jo_vector_fixed *const a, const jo_fixed s, jo_vector_fixed *const result)
Multiply value to vector (using fixed numbers)
Definition: math.h:973