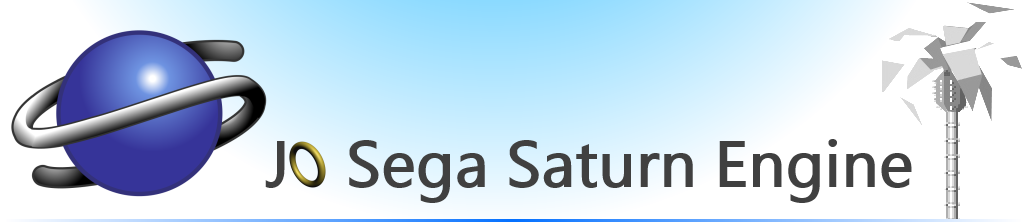 |
Jo Engine
2024.04.28
Jo Sega Saturn Engine
|
Go to the documentation of this file.
36 #ifndef __JO_SOFTWARE_RENDERER_H__
37 # define __JO_SOFTWARE_RENDERER_H__
39 #ifdef JO_COMPILE_WITH_SOFTWARE_RENDERER_SUPPORT
214 const jo_matrix *
const transform_matrix);
236 #endif // JO_COMPILE_WITH_SOFTWARE_RENDERER_SUPPORT
void jo_software_renderer_clear(const jo_software_renderer_gfx *const gfx, const jo_color color)
Clear the buffer with the given color.
void jo_software_renderer_free(jo_software_renderer_gfx *const gfx)
Free the rendering surface.
jo_fixed * depth_buffer
Definition: software_renderer.h:96
@ JO_SR_NO_FACE_CULLING
Definition: software_renderer.h:76
jo_software_renderer_vertex v2
Definition: software_renderer.h:208
Size struct.
Definition: types.h:200
jo_size vram_size
Definition: software_renderer.h:101
jo_color * color_buffer
Definition: software_renderer.h:98
@ JO_SR_FRONT_FACE_CULLING
Definition: software_renderer.h:75
void * vram
Definition: software_renderer.h:100
@ JO_SR_DRAW_FLAT
Definition: software_renderer.h:67
Vector4 for 3D computation using fixed number.
Definition: types.h:165
Vector for 2D computation using fixed number.
Definition: types.h:156
unsigned int color_buffer_size
Definition: software_renderer.h:99
int sprite_id
Definition: software_renderer.h:95
void jo_software_renderer_draw_triangle(const jo_software_renderer_gfx *const gfx, const jo_software_renderer_triangle *const triangle, const jo_matrix *const transform_matrix)
jo_vector4_fixed pos
Definition: software_renderer.h:110
@ JO_SR_DEPTH_GREATER
Definition: software_renderer.h:86
@ JO_SR_DEPTH_IGNORE
Definition: software_renderer.h:82
static __jo_force_inline jo_software_renderer_gfx * jo_software_renderer_create(unsigned short width, unsigned short height, const jo_scroll_screen screen)
Create a full-color rendering surface.
Definition: software_renderer.h:130
jo_software_renderer_draw_mode draw_mode
Definition: software_renderer.h:103
unsigned int depth_buffer_dword_size
Definition: software_renderer.h:97
int sprite_id
Definition: software_renderer.h:209
@ JO_SR_DEPTH_LESS
Definition: software_renderer.h:83
unsigned short jo_color
15 bits color type
Definition: types.h:42
jo_software_renderer_gfx * jo_software_renderer_create_ex(unsigned short width, unsigned short height, const jo_scroll_screen screen, const bool enable_zbuffer)
Create a full-color rendering surface.
jo_software_renderer_face_culling_mode face_culling_mode
Definition: software_renderer.h:104
jo_vector2_fixed uv_texture_mapping
Definition: software_renderer.h:111
#define __jo_force_inline
force inline attribute (and prevent Doxygen prototype parsing bug)
Definition: types.h:39
int jo_fixed
Fixed point Q16.16 number.
Definition: types.h:49
This is the main struct that handle everything for drawing.
Definition: software_renderer.h:93
4x4 MATRIX for 3D computation using fixed number
Definition: types.h:186
jo_software_renderer_face_culling_mode
Face culling mode.
Definition: software_renderer.h:73
@ JO_SR_DRAW_TEXTURED
Definition: software_renderer.h:66
@ JO_SR_DEPTH_NOT_EQUAL
Definition: software_renderer.h:87
@ JO_SR_DRAW_WIREFRAME
Definition: software_renderer.h:68
jo_color color
Definition: software_renderer.h:112
jo_software_renderer_depth_mode
Z-BUFFER Computation mode.
Definition: software_renderer.h:81
@ JO_SR_BACK_FACE_CULLING
Definition: software_renderer.h:74
Triangle definition.
Definition: software_renderer.h:205
jo_software_renderer_vertex v1
Definition: software_renderer.h:207
void jo_software_renderer_draw_pixel2D(const jo_software_renderer_gfx *const gfx, const jo_fixed x, const jo_fixed y, const jo_color color)
Draw a pixel without handling the Z-Buffer.
@ JO_SR_DEPTH_LESS_OR_EQUAL
Definition: software_renderer.h:84
jo_software_renderer_depth_mode depth_mode_testing
Definition: software_renderer.h:102
@ JO_SR_DEPTH_GREATER_OR_EQUAL
Definition: software_renderer.h:85
jo_software_renderer_vertex v0
Definition: software_renderer.h:206
Vertex.
Definition: software_renderer.h:109
static __jo_force_inline void jo_software_renderer_draw_triangle_wireframe(const jo_software_renderer_gfx *const gfx, const jo_software_renderer_triangle *const triangle)
Draw a triangle in wireframe.
Definition: software_renderer.h:220
jo_size clipping_size
Definition: software_renderer.h:94
void jo_software_renderer_draw_pixel3D(const jo_software_renderer_gfx *const gfx, const jo_fixed x, const jo_fixed y, const jo_fixed z, const jo_color color)
Draw a pixel.
@ JO_SR_DEPTH_EQUAL
Definition: software_renderer.h:88
jo_software_renderer_draw_mode
Draw mode.
Definition: software_renderer.h:65
void jo_software_renderer_draw_line3D(const jo_software_renderer_gfx *const gfx, jo_fixed x0, jo_fixed y0, jo_fixed z0, jo_fixed x1, jo_fixed y1, jo_fixed z1, const jo_color color0, const jo_color color1)
Draw a line.
void jo_software_renderer_flush(jo_software_renderer_gfx *const gfx)
Copy the buffer into VDP1/2 VRAM.
jo_scroll_screen
Sega Saturn Scroll Screen Ids.
Definition: types.h:316