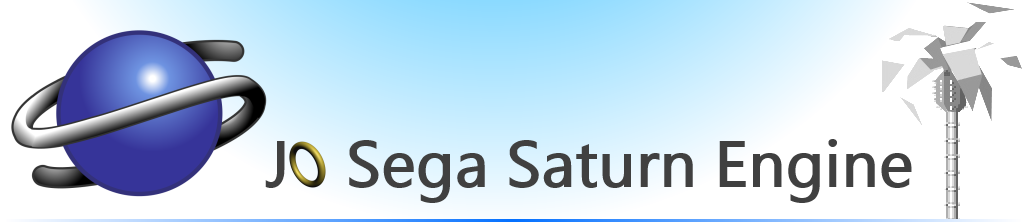 |
Jo Engine
2024.04.28
Jo Sega Saturn Engine
|
Go to the documentation of this file.
35 #ifndef __JO_SPRITES_H__
36 # define __JO_SPRITES_H__
63 void jo_sprite_draw(
const int sprite_id,
const jo_pos3D *
const pos,
const bool centered_style_coordinates,
const bool billboard);
67 void jo_sprite_draw_rotate(
const int sprite_id,
const jo_pos3D *
const pos,
const int angle,
const bool centered_style_coordinates,
const bool billboard);
72 __jo_sprite_pos.
x = x;
73 __jo_sprite_pos.
y = y;
74 __jo_sprite_pos.
z = z;
125 # ifdef JO_COMPILE_WITH_SPRITE_HASHTABLE
230 # define JO_NO_GOURAUD_COLOR (0xc210)
258 # define JO_DEFAULT_BRIGHTNESS (16)
261 # define JO_MAX_BRIGHTNESS (31)
314 # define JO_NO_ZOOM (65535)
458 void jo_sprite_set_clipping_area(
const unsigned int x,
const unsigned int y,
const unsigned int width,
const unsigned int height,
const int depth);
478 return (data[x + y * image_width]);
490 return (data[x + y * image_width]);
static __jo_force_inline int jo_sprite_get_height(const int sprite_id)
Get sprite height.
Definition: sprites.h:519
static __jo_force_inline void jo_sprite_enable_half_transparency(void)
Activate half transparency for every sprite displayed after this call.
Definition: sprites.h:167
static __jo_force_inline void jo_sprite_enable_dark_filter(void)
Activate dark filter for every sprite displayed after this call.
Definition: sprites.h:208
static __jo_force_inline void jo_sprite_draw3D(const int sprite_id, const int x, const int y, const int z)
Display a sprite in 3 dimensional space.
Definition: sprites.h:390
static __jo_force_inline void jo_sprite_change_sprite_scale_xy_fixed(const jo_fixed scale_x, const jo_fixed scale_y)
Change scale for every sprite displayed after this call using fixed number.
Definition: sprites.h:339
static __jo_force_inline void jo_sprite_enable_horizontal_flip(void)
Activate horizontal flip for every sprite displayed after this call.
Definition: sprites.h:292
static __jo_force_inline void jo_sprite_disable_half_transparency(void)
Disable half transparency for every sprite displayed after this call.
Definition: sprites.h:174
static __jo_force_inline void jo_sprite_enable_screen_doors_filter(void)
Activate screen doors filter for every sprite displayed after this call.
Definition: sprites.h:149
void jo_set_gouraud_shading_colors(const jo_color topleft_color, const jo_color topright_color, const jo_color bottomright_color, const jo_color bottomleft_color)
Change Gouraud Shading colors.
static __jo_force_inline void jo_sprite_change_sprite_scale(const float scale)
Change scale for every sprite displayed after this call.
Definition: sprites.h:319
void jo_set_gouraud_shading_brightness(const unsigned char brightness)
Change Gouraud Shading brightness.
#define JO_REMOVE_FLAG(BYTEFIELD, FLAG)
Remove flag in bytefield.
Definition: math.h:330
unsigned short effect
Definition: types.h:267
jo_picture_definition __jo_sprite_pic[JO_MAX_SPRITE]
(internal engine usage)
jo_sprite_attributes __jo_sprite_attributes
Current displayed sprite attribute (internal engine usage)
Texture definition.
Definition: types.h:209
static __jo_force_inline unsigned char jo_sprite_get_pixel_palette_index(const unsigned char *const data, const unsigned int x, const unsigned int y, const unsigned int image_width)
Get a specific pixel palette index from image bytes.
Definition: sprites.h:488
static __jo_force_inline void jo_sprite_disable_screen_doors_filter(void)
Disable screen doors filter for every sprite displayed after this call.
Definition: sprites.h:156
static __jo_force_inline jo_color jo_sprite_get_pixel_color(const jo_color *const data, const unsigned int x, const unsigned int y, const unsigned int image_width)
Get a specific pixel color from image bytes.
Definition: sprites.h:476
static __jo_force_inline void jo_sprite_enable_clipping(bool outside)
Activate sprite clipping for every sprite displayed after this call.
Definition: sprites.h:438
static __jo_force_inline void jo_sprite_change_sprite_scale_xy(const float scale_x, const float scale_y)
Change scale for every sprite displayed after this call.
Definition: sprites.h:329
unsigned int color_table_index
Definition: types.h:269
static __jo_force_inline void jo_sprite_draw3D_and_rotate(const int sprite_id, const int x, const int y, const int z, const int angle)
Display a sprite in 3 dimensional space.
Definition: sprites.h:377
void jo_sprite_draw(const int sprite_id, const jo_pos3D *const pos, const bool centered_style_coordinates, const bool billboard)
(internal engine usage)
#define JO_MULT_BY_256(X)
Multiply a variable by 256.
Definition: math.h:108
jo_fixed fixed_scale_y
Definition: types.h:272
int z
Definition: types.h:72
static __jo_force_inline void jo_sprite_disable_shadow_filter(void)
Disable shadow filter for every sprite displayed after this call.
Definition: sprites.h:197
static __jo_force_inline void jo_sprite_disable_horizontal_flip(void)
Disable horizontal flip for every sprite displayed after this call.
Definition: sprites.h:299
unsigned short jo_color
15 bits color type
Definition: types.h:42
static __jo_force_inline int jo_sprite_count(void)
Get sprite count.
Definition: sprites.h:545
unsigned short direction
Definition: types.h:268
Picture definition.
Definition: types.h:218
8 bits image struct
Definition: types.h:234
int jo_sprite_add(const jo_img *const img)
Add a sprite.
static __jo_force_inline void jo_sprite_free_all(void)
Free all sprites.
Definition: sprites.h:120
#define __jo_force_inline
force inline attribute (and prevent Doxygen prototype parsing bug)
Definition: types.h:39
int jo_fixed
Fixed point Q16.16 number.
Definition: types.h:49
#define JO_COLOR_Transparent
Transparent color.
Definition: colors.h:87
int y
Definition: types.h:71
static __jo_force_inline void jo_sprite_disable_vertical_flip(void)
Disable vertical flip for every sprite displayed after this call.
Definition: sprites.h:286
#define JO_ADD_FLAG(BYTEFIELD, FLAG)
Add flag in bytefield.
Definition: math.h:324
void jo_sprite_free_from(const int sprite_id)
Free all sprites from the given sprite_id.
static __jo_force_inline void jo_sprite_disable_clipping(void)
Disable sprite clipping for every sprite displayed after this call.
Definition: sprites.h:445
static __jo_force_inline void jo_sprite_set_palette(int palette_id)
Change palette for every 8 bits sprite displayed after this call.
Definition: sprites.h:365
void jo_sprite_set_clipping_area(const unsigned int x, const unsigned int y, const unsigned int width, const unsigned int height, const int depth)
Set user clipping area.
static __jo_force_inline void jo_sprite_disable_dark_filter(void)
Disable dark filter for every sprite displayed after this call.
Definition: sprites.h:215
static __jo_force_inline void jo_sprite_enable_vertical_flip(void)
Activate vertical flip for every sprite displayed after this call.
Definition: sprites.h:279
int jo_sprite_replace(const jo_img *const img, const int sprite_id)
Replace a sprite.
static __jo_force_inline void jo_sprite_draw3D2(const int sprite_id, const int x, const int y, const int z)
Display a sprite in 3 dimensional space.
Definition: sprites.h:417
static __jo_force_inline void jo_sprite_disable_gouraud_shading(void)
Disable Gouraud Shading for every sprite displayed after this call.
Definition: sprites.h:252
static __jo_force_inline int jo_sprite_get_width(const int sprite_id)
Get sprite width.
Definition: sprites.h:510
static __jo_force_inline void jo_sprite_restore_sprite_scale(void)
Restore default scale for every sprite displayed after this call.
Definition: sprites.h:347
void jo_sprite_draw_rotate(const int sprite_id, const jo_pos3D *const pos, const int angle, const bool centered_style_coordinates, const bool billboard)
(internal engine usage)
3D position
Definition: types.h:69
int x
Definition: types.h:70
static __jo_force_inline void * jo_sprite_get_raw_data(const int sprite_id)
Get sprite raw image contents.
Definition: sprites.h:528
jo_fixed fixed_scale_x
Definition: types.h:271
static __jo_force_inline void jo_sprite_draw3D_and_rotate2(const int sprite_id, const int x, const int y, const int z, const int angle)
Display a sprite in 3 dimensional space.
Definition: sprites.h:404
static __jo_force_inline void __internal_jo_sprite_set_position3D(const int x, const int y, const int z)
Internal usage don't use it.
Definition: sprites.h:69
static __jo_force_inline bool jo_sprite_is_pixel_transparent(const jo_color *const data, const unsigned int x, const unsigned int y, const unsigned int image_width)
Get if a specific pixel is transparent (JO_COLOR_Transparent) in image bytes.
Definition: sprites.h:501
unsigned int clipping
Definition: types.h:273
Sprite attributes.
Definition: types.h:266
int jo_sprite_name2id(const char *const filename)
Retrive the Sprite Id from filename based on the four first character in the filename.
static __jo_force_inline void jo_sprite_enable_shadow_filter(void)
Activate shadow filter for every sprite displayed after this call.
Definition: sprites.h:190
int jo_get_last_sprite_id(void)
Get last Sprite Id.
static __jo_force_inline jo_fixed jo_float2fixed(const float x)
Convert float to jo engine fixed (avoid usage of GCC Soft Float)
Definition: math.h:433
void jo_sprite_draw_4p_fixed(const int sprite_id, const jo_pos2D_fixed *const four_points, const jo_fixed z, const bool centered_style_coordinates)
2D position using fixed number
Definition: types.h:60
int jo_sprite_usage_percent(void)
Get sprite memory usage.
int jo_sprite_add_8bits_image(const jo_img_8bits *const img)
Add a 8 bits sprite.
15 bits image struct
Definition: types.h:226
jo_texture_definition __jo_sprite_def[JO_MAX_SPRITE]
(internal engine usage)
#define JO_NO_ZOOM
Value used to keep the original scale.
Definition: sprites.h:314
static __jo_force_inline void jo_sprite_enable_gouraud_shading(void)
Activate Gouraud Shading for every sprite displayed after this call.
Definition: sprites.h:245