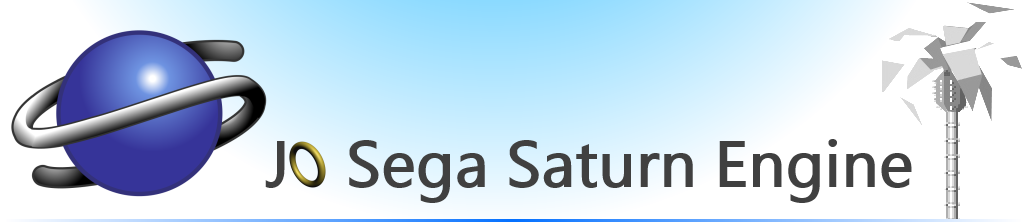 |
Jo Engine
2024.04.28
Jo Sega Saturn Engine
|
Go to the documentation of this file.
35 #ifndef __JO_TOOLS_H__
36 # define __JO_TOOLS_H__
44 #define JO_FIXED_TV_WIDTH JO_INT_TO_FIXED(JO_TV_WIDTH)
46 #define JO_FIXED_TV_HEIGHT JO_INT_TO_FIXED(JO_TV_HEIGHT)
54 return ((((value) >> 8) & 0xff) | (((value) & 0xff) << 8));
63 return ((((value) >> 8) & 0xff) | (((value) & 0xff) << 8));
72 return ((((value) & 0xff000000) >> 24) | (((value) & 0x00ff0000) >> 8) | (((value) & 0x0000ff00) << 8) | (((value) & 0x000000ff) << 24));
81 return ((((value) & 0xff000000) >> 24) | (((value) & 0x00ff0000) >> 8) | (((value) & 0x0000ff00) << 8) | (((value) & 0x000000ff) << 24));
90 int sprintf(
char* str,
const char* format, ...);
94 #define jo_printf_debug(X, Y, fmt, args...) \
95 jo_printf(X, Y, "%s(%s)#%d : " fmt , \
96 __FILE__, __FUNCTION__, __LINE__, ## args)
105 #ifdef JO_COMPILE_WITH_PRINTF_SUPPORT
107 #if !JO_COMPILE_USING_SGL
108 extern unsigned char __jo_printf_current_palette_index;
116 void jo_print(
int x,
int y,
char * str);
123 # define jo_printf(X, Y, ...) do {sprintf(__jo_sprintf_buf, __VA_ARGS__); jo_print(X, Y, __jo_sprintf_buf); } while(0)
131 # define jo_printf_with_color(X, Y, COLOR_INDEX, ...) do { jo_set_printf_color_index(COLOR_INDEX); jo_printf(X, Y, __VA_ARGS__); } while(0)
136 static __jo_force_inline void jo_set_printf_color_index(
const unsigned char index)
138 #if JO_COMPILE_USING_SGL
141 __jo_printf_current_palette_index = index;
148 void jo_clear_screen(
void);
154 void jo_clear_screen_line(
const int y);
156 #endif // JO_COMPILE_WITH_PRINTF_SUPPORT
166 #if JO_COMPILE_USING_SGL
167 slDMACopy(src, dest, size);
169 slDMACopy(src, dest, size);
175 # define JO_NULL ((void *)0)
179 # define JO_UNUSED_ARG(ARG) ((void)ARG)
187 return c ==
'\n' || c ==
'\r' || c ==
'\t' || c ==
' ';
196 return (
int)str[0] | (((int)str[1]) << 8) | (((
int)str[2]) << 16) | (((int)str[3]) << 24);
260 int jo_strcmp(
const char * restrict p1,
const char * restrict p2);
277 bool jo_endwith(
const char * restrict str,
const char * restrict end);
285 void jo_memset(
const void *
const restrict ptr,
const int value,
unsigned int num);
310 void jo_map_to_vram(
unsigned short * restrict data,
unsigned short * restrict vram_addr,
311 unsigned short suuj,
unsigned short suui,
unsigned short palnum,
unsigned int mapoff);
320 while (size-- > 0) *(vram_addr++) = *(data++);
#define JO_PRINTF_BUF_SIZE
Max character in available in jo_printf()
Definition: conf.h:165
#define __jo_force_inline
force inline attribute (and prevent Doxygen prototype parsing bug)
Definition: types.h:39