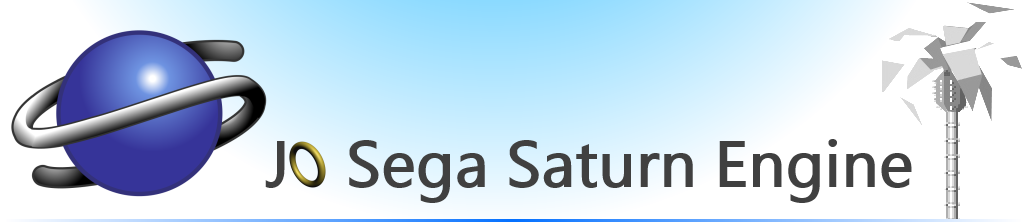 |
Jo Engine
2024.04.28
Jo Sega Saturn Engine
|
Go to the documentation of this file.
35 #ifndef __JO_VOXEL_H__
36 # define __JO_VOXEL_H__
38 #ifdef JO_COMPILE_WITH_SOFTWARE_RENDERER_SUPPORT
222 #endif // JO_COMPILE_WITH_SOFTWARE_RENDERER_SUPPORT
jo_fixed ply
Definition: voxel.h:46
jo_fixed height
Definition: voxel.h:59
static __jo_force_inline void jo_voxel_move_backward(jo_voxel *const voxel_data, const jo_fixed distance)
Move camera backward (camera.x & camera.y)
Definition: voxel.h:196
void jo_software_renderer_clear(const jo_software_renderer_gfx *const gfx, const jo_color color)
Clear the buffer with the given color.
int map_width_minus_1
Definition: voxel.h:49
jo_fixed plx
Definition: voxel.h:45
Voxel main definition.
Definition: voxel.h:68
static __jo_force_inline void jo_voxel_rotate_left(jo_voxel *const voxel_data, const jo_fixed angle_rad)
Rotate camera on the left (camera.angle)
Definition: voxel.h:206
jo_img_8bits terrain_img
Definition: voxel.h:72
jo_palette height_pal
Definition: voxel.h:71
bool use_zbuffer
Definition: voxel.h:75
static __jo_force_inline void jo_voxel_move_up(jo_voxel *voxel_data, const jo_fixed distance)
Increase camera height (camera.height)
Definition: voxel.h:168
static __jo_force_inline void jo_voxel_redraw_and_flush(jo_voxel *const voxel_data)
Draw voxels and flush the buffer.
Definition: voxel.h:110
jo_fixed angle
Definition: voxel.h:62
jo_fixed scale
Definition: voxel.h:56
Palette contents struct.
Definition: types.h:250
jo_fixed jo_fixed_cos(jo_fixed rad)
Fast cosinus computation using fixed number.
__jo_voxel_computation_cache __cache
Definition: voxel.h:76
jo_voxel_camera camera
Definition: voxel.h:74
static __jo_force_inline void jo_voxel_move_down(jo_voxel *const voxel_data, const jo_fixed distance)
Decrease camera height (camera.height)
Definition: voxel.h:177
jo_fixed zfar
Definition: voxel.h:61
8 bits image struct
Definition: types.h:234
jo_fixed y
Definition: voxel.h:58
void jo_voxel_run(jo_voxel *const voxel_data)
Draw voxels to gfx.
#define __jo_force_inline
force inline attribute (and prevent Doxygen prototype parsing bug)
Definition: types.h:39
int jo_fixed
Fixed point Q16.16 number.
Definition: types.h:49
jo_software_renderer_gfx * gfx
Definition: voxel.h:69
This is the main struct that handle everything for drawing.
Definition: software_renderer.h:93
static __jo_force_inline void jo_voxel_move_forward(jo_voxel *const voxel_data, const jo_fixed distance)
Move camera forward (camera.x & camera.y)
Definition: voxel.h:186
jo_palette terrain_pal
Definition: voxel.h:70
jo_fixed px
Definition: voxel.h:47
jo_fixed x
Definition: voxel.h:57
static __jo_force_inline void jo_voxel_decrease_view_distance(jo_voxel *const voxel_data, const jo_fixed distance)
Decrease view distance (camera.zfar)
Definition: voxel.h:140
#define JO_COLOR_Black
Definition: colors.h:57
jo_fixed horizon
Definition: voxel.h:60
jo_img_8bits height_img
Definition: voxel.h:73
Voxel computation cache (internal engine usage)
Definition: voxel.h:44
jo_fixed py
Definition: voxel.h:48
static __jo_force_inline void jo_voxel_increase_view_distance(jo_voxel *const voxel_data, const jo_fixed distance)
Increase view distance (camera.zfar)
Definition: voxel.h:130
static __jo_force_inline void jo_voxel_horizon_up(jo_voxel *const voxel_data, const jo_fixed distance)
Increase horizon height (camera.horizon)
Definition: voxel.h:150
jo_fixed jo_fixed_mult(jo_fixed x, jo_fixed y)
Multiply to fixed number.
void jo_voxel_do_angle_computation(jo_voxel *const voxel_data)
Do computation linked to camera angle.
jo_fixed jo_fixed_sin(jo_fixed rad)
Fast sinus computation using fixed number.
static __jo_force_inline void jo_voxel_rotate_right(jo_voxel *const voxel_data, const jo_fixed angle_rad)
Rotate camera on the right (camera.angle)
Definition: voxel.h:216
static __jo_force_inline void jo_voxel_horizon_down(jo_voxel *const voxel_data, const jo_fixed distance)
Decrease horizon height (camera.horizon)
Definition: voxel.h:159
void jo_voxel_redraw(jo_voxel *const voxel_data)
Draw voxels to gfx.
Voxel camera definition.
Definition: voxel.h:55
void jo_software_renderer_flush(jo_software_renderer_gfx *const gfx)
Copy the buffer into VDP1/2 VRAM.