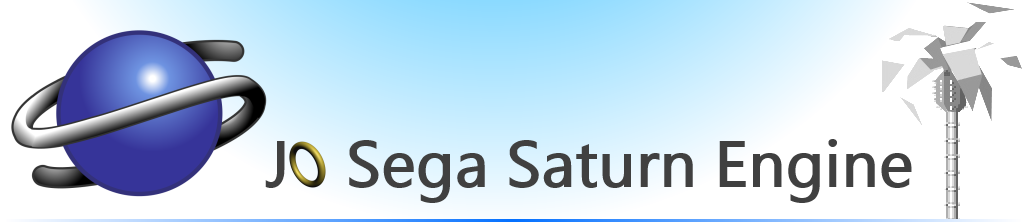 |
Jo Engine
2024.04.28
Jo Sega Saturn Engine
|
Go to the documentation of this file.
36 #ifndef __JO_INPUT_H__
37 # define __JO_INPUT_H__
39 # define JO_INPUT_MAX_DEVICE (12)
42 #define PER_ID_NightsPad 0x16
44 #if JO_COMPILE_USING_SGL
46 # define __JO_KEY_PRESSED(PORT, KEY) ((jo_inputs[PORT].data & KEY) == 0)
48 # define __JO_KEY_PRESSED(PORT, KEY) ((jo_inputs[PORT].pressed & KEY) == KEY)
51 #if !JO_COMPILE_USING_SGL
59 unsigned short pressed;
60 unsigned short on_keydown;
61 unsigned short on_keyup;
73 #if JO_COMPILE_USING_SGL
169 return (
jo_inputs[port].
id != PER_ID_NotConnect);
229 #if JO_COMPILE_USING_SGL
230 return ((
unsigned short)
jo_inputs[port].data);
232 return ((
unsigned short)
jo_inputs[port].pressed);
243 #if JO_COMPILE_USING_SGL
244 return ((
jo_inputs[port].push & key) == 0);
246 return ((
jo_inputs[port].on_keydown & key) == key);
250 #if !JO_COMPILE_USING_SGL
259 return ((
jo_inputs[port].on_keyup & key) == key);
263 #if JO_COMPILE_USING_SGL
@ RIGHT
Definition: types.h:305
@ UP_RIGHT
Definition: types.h:309
#define JO_DIV_BY_65536(X)
Devide a variable by 65536.
Definition: math.h:192
@ UP_LEFT
Definition: types.h:308
@ DOWN_RIGHT
Definition: types.h:311
@ UP
Definition: types.h:306
#define JO_MULT_BY_65536(X)
Multiply a variable by 65536.
Definition: math.h:138
#define __jo_force_inline
force inline attribute (and prevent Doxygen prototype parsing bug)
Definition: types.h:39
@ NONE
Definition: types.h:303
@ DOWN
Definition: types.h:307
jo_8_directions
8 directions definition
Definition: types.h:302
@ DOWN_LEFT
Definition: types.h:310
@ LEFT
Definition: types.h:304