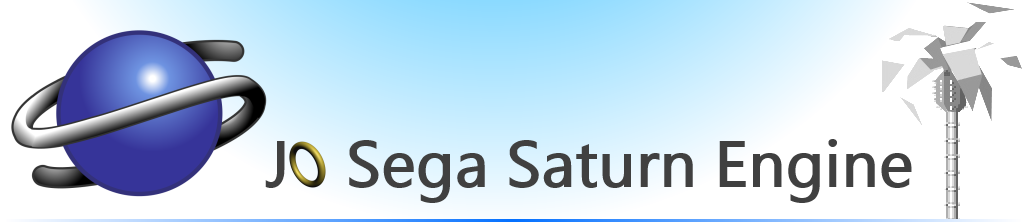 |
Jo Engine
2024.04.28
Jo Sega Saturn Engine
|
Go to the documentation of this file.
45 # define __JO_VDP2_H__
53 int sprintf(
char* str,
const char* format, ...);
79 #ifndef JO_COMPILE_WITH_PRINTF_SUPPORT
93 # define jo_nbg2_printf(X, Y, ...) do {sprintf(__jo_sprintf_buf, __VA_ARGS__); jo_nbg2_print(X, Y, __jo_sprintf_buf); } while(0)
121 # define jo_nbg3_printf(X, Y, ...) do {sprintf(__jo_sprintf_buf, __VA_ARGS__); jo_nbg3_print(X, Y, __jo_sprintf_buf); } while(0)
157 #ifndef JO_COMPILE_WITH_PRINTF_SUPPORT
214 #if JO_COMPILE_USING_SGL
228 #if JO_COMPILE_USING_SGL
229 slZoomNbg1(toFIXED(width_factor), toFIXED(height_factor));
254 #if JO_COMPILE_USING_SGL
268 #if JO_COMPILE_USING_SGL
269 slZoomNbg0(toFIXED(width_factor), toFIXED(height_factor));
330 *(((
unsigned short *)VDP2_VRAM_A0) + x + y *
JO_VDP2_WIDTH) = color;
398 #if JO_COMPILE_USING_SGL
405 slCurRpara(RA);
if (use_scroll_format_matrix) slScrMatConv(); slScrMatSet();
413 slCurRpara(RB);
if (use_scroll_format_matrix) slScrMatConv(); slScrMatSet();
427 #if JO_COMPILE_USING_SGL
438 slScrMosaicOn(screens);
472 # define JO_NBG1_SCROLL_TABLE_SIZE (512)
491 # define JO_NBG0_SCROLL_TABLE_SIZE (512)
void jo_vdp2_replace_rbg0_plane_a_8bits_image(jo_img_8bits *img, bool vertical_flip)
Replace plane A image.
static __jo_force_inline void jo_vdp2_move_nbg0(const int x, const int y)
Move NBG0 (scrolling)
Definition: vdp2.h:252
void jo_enable_screen_transparency(const jo_scroll_screen screen, const unsigned short transparency_level)
Enable screen transparency.
#define JO_VDP2_ZMYIN0
Definition: sega_saturn.h:300
static __jo_force_inline void jo_vdp2_draw_bitmap_nbg1_square(const int x, const int y, const short width, const short height, const jo_color color)
Draw a square on NBG1.
Definition: vdp2.h:315
void jo_nbg2_clear(void)
Clear NBG2.
#define JO_VDP2_SCXIN1
Definition: sega_saturn.h:303
static __jo_force_inline void jo_vdp2_zoom_nbg1f(const float width_factor, const float height_factor)
Zoom NBG1 width and height independently.
Definition: vdp2.h:226
void jo_vdp2_set_nbg2_8bits_font(jo_img_8bits *img, char *mapping, int palette_id, bool vertical_flip, bool enabled)
Set 8 bits NBG2 font image.
static __jo_force_inline void jo_vdp2_zoom_nbg1(const float factor)
Zoom NBG1.
Definition: vdp2.h:243
void jo_set_displayed_screens(const jo_scroll_screen scroll_screen_flags)
Set displayed screens.
int * jo_vdp2_enable_nbg1_line_scroll(void)
Enable NBG1 horizontal line scroll effect.
#define JO_VDP2_ZMXDN1
Definition: sega_saturn.h:308
int sprintf(char *str, const char *format,...)
sprintf prototypes
#define JO_MOD_POW2(N, M)
Fast modulo of a power of 2.
Definition: math.h:220
void jo_vdp2_set_nbg1_8bits_image(jo_img_8bits *img, int palette_id, bool vertical_flip)
Add 8 bits NBG1 image.
#define JO_VDP2_ZMYIN1
Definition: sega_saturn.h:309
#define JO_VDP2_ZMYDN1
Definition: sega_saturn.h:310
#define JO_MULT_BY_65536(X)
Multiply a variable by 65536.
Definition: math.h:138
static __jo_force_inline void jo_vdp2_draw_rbg0_plane_b(const bool use_scroll_format_matrix)
Draw plane A.
Definition: vdp2.h:411
#define JO_VDP2_SCYIN1
Definition: sega_saturn.h:305
int * jo_vdp2_enable_nbg0_line_scroll(void)
Enable NBG0 horizontal line scroll effect.
void jo_vdp2_compute_nbg1_line_scroll(unsigned short offset)
Compute NBG1 horizontal line scroll effect using specific offset.
void jo_vdp2_disable_nbg1_line_scroll(void)
Disable NBG1 horizontal line scroll effect.
void jo_set_default_background_color(const jo_color background_color)
Set default background color.
void jo_vdp2_set_nbg3_8bits_font(jo_img_8bits *img, char *mapping, int palette_id, bool vertical_flip, bool enabled)
Set 8 bits NBG3 font image.
char __jo_sprintf_buf[JO_PRINTF_BUF_SIZE]
Internal sprintf buffer.
void jo_vdp2_draw_bitmap_nbg1_line(int x0, int y0, int x1, int y1, const jo_color color)
Draw a NBG1 line using Bresenham's line algorithm.
void jo_vdp2_set_nbg3_8bits_image(jo_img_8bits *img, int palette_id, bool vertical_flip, bool enabled)
Set 8 bits NBG3 image.
void jo_vdp2_disable_rbg0(void)
Disable 3D planes.
unsigned short jo_color
15 bits color type
Definition: types.h:42
static __jo_force_inline void jo_vdp2_zoom_nbg0f(const float width_factor, const float height_factor)
Zoom NBG0 width and height independently.
Definition: vdp2.h:266
static __jo_force_inline void jo_vdp2_move_nbg1(const int x, const int y)
Move NBG1 (scrolling)
Definition: vdp2.h:212
void jo_vdp2_set_nbg0_8bits_image(jo_img_8bits *img, int palette_id, bool vertical_flip, bool enabled)
Set 8 bits NBG0 image.
#define JO_DIV_BY_32768(X)
Devide a variable by 32768.
Definition: math.h:186
#define JO_PRINTF_BUF_SIZE
Max character in available in jo_printf()
Definition: conf.h:165
8 bits image struct
Definition: types.h:234
#define __jo_force_inline
force inline attribute (and prevent Doxygen prototype parsing bug)
Definition: types.h:39
void jo_nbg3_clear(void)
Clear NBG3.
#define JO_VDP2_ZMXIN1
Definition: sega_saturn.h:307
#define JO_VDP2_WIDTH
VDP2 Background bitmap width.
Definition: conf.h:159
void jo_vdp2_replace_rbg0_plane_b_8bits_image(jo_img_8bits *img, bool vertical_flip)
Replace plane B image.
static __jo_force_inline void jo_vdp2_draw_rbg0_plane_a(const bool use_scroll_format_matrix)
Draw plane A.
Definition: vdp2.h:403
void jo_vdp2_compute_nbg0_line_scroll(unsigned short offset)
Compute NBG0 horizontal line scroll effect using specific offset.
void jo_nbg2_print(int x, int y, char *str)
NBG2 Print implementation.
#define JO_VDP2_SCXIN0
Definition: sega_saturn.h:294
void jo_vdp2_set_nbg1_image(const jo_img *const img, const unsigned short left, const unsigned short top)
Set NBG1 bitmap image.
#define JO_VDP2_ZMYDN0
Definition: sega_saturn.h:301
void jo_disable_all_screens_transparency(void)
Disable all screens transparency.
void jo_vdp2_set_rbg0_plane_a_8bits_image(jo_img_8bits *img, int palette_id, bool repeat, bool vertical_flip)
Setup plane A.
void jo_vdp2_set_nbg2_8bits_image(jo_img_8bits *img, int palette_id, bool vertical_flip, bool enabled)
Set 8 bits NBG2 image.
static __jo_force_inline void jo_vdp2_zoom_nbg0(const float factor)
Zoom NBG0.
Definition: vdp2.h:283
static __jo_force_inline void jo_vdp2_put_pixel_bitmap_nbg1(const int x, const int y, const jo_color color)
Put pixel in NBG1 using color.
Definition: vdp2.h:328
void jo_nbg3_print(int x, int y, char *str)
NBG3 Print implementation.
void jo_vdp2_clear_bitmap_nbg1(const jo_color color)
Clear NBG1 bitmap.
static __jo_force_inline void jo_vdp2_put_pixel_bitmap_nbg1_rgb(const int x, const int y, unsigned char r, unsigned char g, unsigned char b)
Put pixel in NBG1 using composite color.
Definition: vdp2.h:340
static __jo_force_inline void jo_enable_screen_mozaic(const jo_scroll_screen screens, const short x, const short y)
Enable mozaic effect for scroll screen.
Definition: vdp2.h:435
static __jo_force_inline void jo_disable_all_screen_mozaic(void)
Disable mozaic effect for all scroll screen.
Definition: vdp2.h:443
#define JO_VDP2_SCYIN0
Definition: sega_saturn.h:296
static __jo_force_inline jo_fixed jo_float2fixed(const float x)
Convert float to jo engine fixed (avoid usage of GCC Soft Float)
Definition: math.h:433
#define JO_VDP2_ZMXDN0
Definition: sega_saturn.h:299
void jo_vdp2_enable_rbg0(void)
Enable 3D planes.
15 bits image struct
Definition: types.h:226
#define JO_VDP2_ZMXIN0
Definition: sega_saturn.h:298
void jo_vdp2_disable_nbg0_line_scroll(void)
Disable NBG0 horizontal line scroll effect.
void jo_vdp2_set_rbg0_plane_b_8bits_image(jo_img_8bits *img, int palette_id, bool repeat, bool vertical_flip)
Setup plane B.
jo_scroll_screen
Sega Saturn Scroll Screen Ids.
Definition: types.h:316